Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial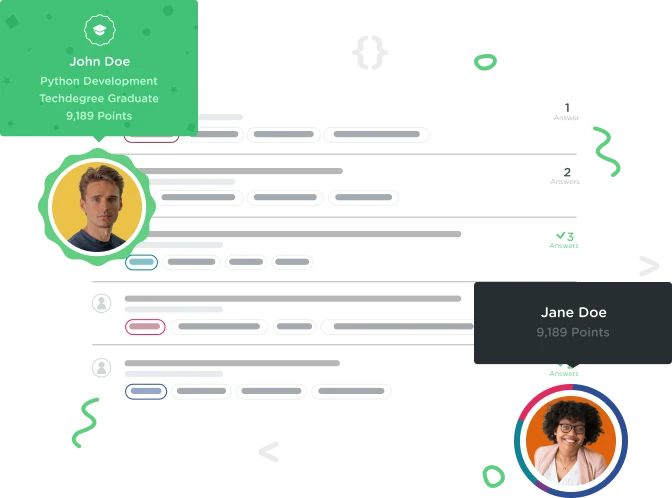
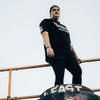
Michael Davis
6,992 PointsCan someone help me understand what I'm doing wrong
I'm trying to figure out why my code isn't working. In my mind it makes sense but it says "unexpected identifier"
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
get major() {
return this._major
}
set major(major) {
this._major = major
if (this.level !== 'Senior' || this.level !== 'Junior') {
return this._major;
}else {
this._major = 'none';
}
}
var student = new Student(3.9, 60);
1 Answer
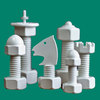
Steven Parker
231,275 PointsThe braces are unbalanced, there seems to be a missing closing brace for the method.
A few extra hints:
- check the comparisons and logic to be sure they are doing what you want
- setters don't need to return anything
- you don't need to create a getter for this challenge
Michael Davis
6,992 PointsMichael Davis
6,992 PointsI'm still confused. Are you saying it should look more like this?
set major(major) { this._major = major if (this.level !== 'Senior' || this.level !== 'Junior') { } else { this._major = 'none'; } }
Steven Parker
231,275 PointsSteven Parker
231,275 PointsInstead of an empty block with an "else", you could just have a normal "if" where comparisons (and logic) check for the condition(s) where you want to reassign the backing variable.