Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial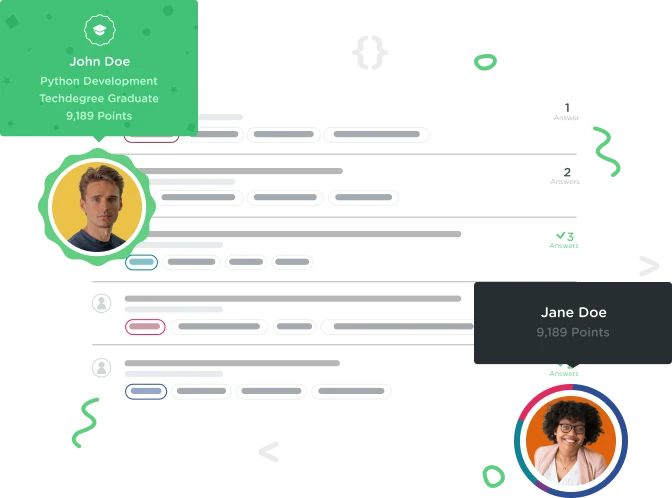

Dennis Zern
813 PointsCan someone help me with these IllegegalArgumentExceptions? With a challenge from Java objects.
Challenge Task 1 of 1
Example.java contains a program using the GoKart class we have been building (included for you to reference).
Protect the call to kart.drive by handling the IllegalArgumentException that is thrown when not enough battery remains. Print out the message from the exception to the screen as you catch the exception. Important: In each task of this code challenge, the code you write should be added to the code from the previous task. Restart Preview Get Help Check Work Example.java GoKart.java
1 class Example { 2 ā 3 public static void main(String[] args) { 4 GoKart kart = new GoKart("purple"); 5 if (kart.isBatteryEmpty()) { 6 System.out.println("The battery is empty"); 7 } 8 kart.drive(42); 9 } 10
11
12 ā 13 } 14 ā
WHAT am i supposed to write here? Explain why please if so.
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive(42);
}
}
class GoKart {
public static final int MAX_BARS = 8;
private int barCount;
private String color;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
lapsDriven += laps;
barCount -= laps;
}
}
7 Answers
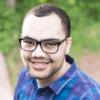
Philip Gales
15,193 PointsDon't change GoKart.java. They want you to protect kart.drive(42) by wrapping it in a try-catch block.
Remember, they look like this:
try {
} catch(Exception e) {
}
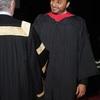
Mohammed AlShaiban
6,455 PointsFirst, you need to wrap kart.drive(42) it in a try-catch block., then in the catch, you need to output a message using getMessage() method in the Throwable class
public String getMessage()
Returns the detail message string of this throwable.
Returns:
the detail message string of this Throwable instance (which may be null).
try {
kart.drive(42);
} catch(IllegalArgumentException iae) {
System.out.println(iae.getMessage());
}
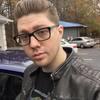
Cameron Hyatt
5,948 PointsSystem.out.println( "%s", iae.getMessage());
System.out.println(iae.getMessage());
In case anyone is getting weird "Can't find the symbol" errors for the getMessage method, make sure you didn't do the same mistake I did, which is the first line. Removing the "%s" bit and leaving it just as the method works perfectly. I had assumed I need to have a string for it to replace in order for it to print at all.
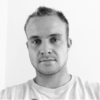
peterdemain
8,001 Pointsclass Example {
public static void main(String[] args) { GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } try { kart.drive(42); } catch(IllegalArgumentException iae) { System.out.println(iae.getMessage()); } }
}
Thank you :D

chase singhofen
3,811 Pointsi used this
System.out.println( iae ); }
i tried using the .getMessage but got syntax error idk why??
also, i dont understand why we dont use quotation marks in the print out message? we do this in other print out lines.

Max Meijer
3,048 Pointsbecaus you used a ; you can leave that a little a late but yeah xD
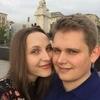
christopher Pettersen
6,900 PointsHi there!
I have tried every thing, and it is probably something dumb that i dont see. i constantly get syntax error and i cant understand why?
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
try {
kart.drive(42);
} catch(IllegalArgumentException iae) {
System.out.println(iae.getMessage());
}
}

Sarah Wisner
644 Pointschristopher Pettersen It looks like you are missing the closing bracket for the "if" statement above the new code. Try putting a "}" before the try/catch statements.

Markus Amon
709 PointsThis is what it should look like ^^
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(42);
} catch (IllegalArgumentException iae) {
System.out.printf("%s %n",
iae.getMessage());
}
}
}

Bryan Castillo
2,484 PointsThis was my code and it wont work for idk what reason it claims that it cant find iae symbol in my printf method.
try { kart.drive(42); } catch(IllegalArgumentException iae) { System.out.println("NOT ENOUGH RICE");} System.out.printf("THE ERROR WAS %S",iae.getMessage());
} }

Ashish Shah
1,468 PointsDid you got answer?
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsWhen you see the phrase 'included for you to reference', know that you will not change it, and that the answer will probably contain a method from the class they included for you to reference.
In this case, we did not need to reference the class since they told us what error to expect.
Dennis Zern
813 PointsDennis Zern
813 PointsWhere am i supposed to write it?
Philip Gales
15,193 PointsPhilip Gales
15,193 PointsYou are supposed to wrap the try-catch around the kart.drive(42).
They even give you the exception to catch. 'IllegalArgumentException' and tell you to display the exception. So lets replace my generic exception and display the new one.
Ashish Shah
1,468 PointsAshish Shah
1,468 PointsThis is showing syntax error.
class Example {
public static void main(String[] args) GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } try {
kart.drive(42);
} catch(IllegalArgumentException iae) {
Stewart Mandla Kohlisa
11,737 PointsStewart Mandla Kohlisa
11,737 Pointsmay you pliz post the entire code code coz um dont know what um doing wrong