Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial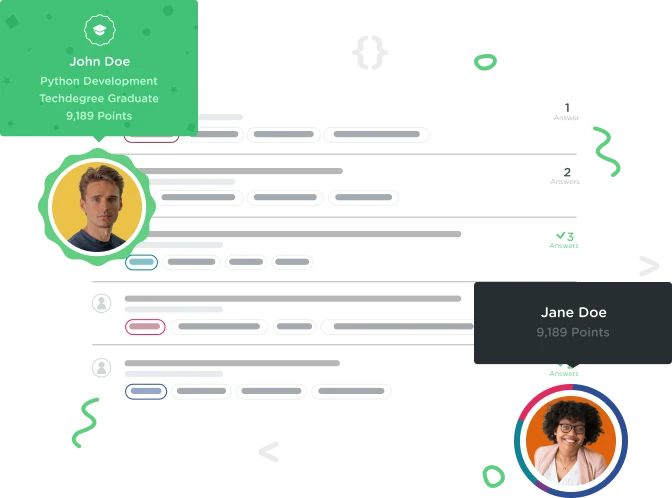

Andrei Oprescu
9,547 PointsCan someone help me with this challenge?
Hi!
I am doing this challenge which asks me this:
Let's write some functions to explore set math a bit more. We're going to be using this COURSES dict in all of the examples. Don't change it, though!
So, first, write a function named covers that accepts a single parameter, a set of topics. Have the function return a list of courses from COURSES where the supplied set and the course's value (also a set) overlap.
For example, covers({"Python"}) would return ["Python Basics"].
My code that I used for this challenge is at the bottom of this question.
Can someone tell me what I did wrong?
Thanks!
Andrei
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(topics):
return topics.intersection(COURSES)
6 Answers
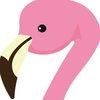
Dave StSomeWhere
19,870 PointsHi Andrei,
Two issues I noticed:
-
your statement below returns a set, not a list as per the instructions.
return topics.intersection(COURSES)
COURSES is a set of sets, so doing the intersection with COURSES does not return anything. You need to iterate through the sets and sets do not support indexes. I used a for loop, do you know how to loop through COURSES and then return each course including the topic as a list item?

Andrei Oprescu
9,547 PointsHi Dave!
I have followed your instructions and came up with this code:
def covers(topics):
result = []
for key, value in COURSES.items():
if topics.intersection(value) is True:
result.append(key)
return result
I tried to run it in the workspaces and it gave me no result. Can you please suggest what I did wrong this time?
Thanks!
Andrei
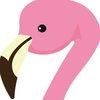
Dave StSomeWhere
19,870 PointsIf that is the code you tried in workspaces you would still need to define a function to return a value and I don't see where you are setting the value for topics.
For debugging just try print() to get it worked out. The only issue I see is the "is True" on the if statement - doing just the intersection with result in a True or False result and you can verify you are getting a result with prints (or define the whole thing and do the print on result after.
if topics.intersection(value):
print(key)
print(value)
The loop and append look correct.

Andrei Oprescu
9,547 PointsHi Dave!
I have followed your advice and it was very useful. When I tried this code in the workspace it printed out all of the tracks that had a specific topic in it:
def covers(topics): result = [] for key, value in COURSES.items(): if topics.intersection(value): print(key) return result
although when i tried this code:
def covers(topics): result = [] for key, value in COURSES.items(): if topics.intersection(value): result.append(key) return result
I got no topics.
can you tell me why this might be?
Thanks!
Andrei
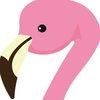
Dave StSomeWhere
19,870 PointsAre you sure you are indenting correctly?
When I run your code as per below, it is happy and works properly:
def covers(topics):
result = []
for key, value in COURSES.items():
if topics.intersection(value):
result.append(key)
return result
print(str(covers({"Python"})))

Andrei Oprescu
9,547 PointsHi Dave!
Sorry! It was my mistake since when I tried out my code I just called my function, but did not also print it! :)
Thank you!
Andrei

Tor Ivan Boine
4,057 PointsDave varmutant, I tried your code in IDLE, and it fails
>>> covers('Python')
Traceback (most recent call last):
File "<pyshell#119>", line 1, in <module>
covers('Python')
File "H:\Dropbox\3 Programmering\Python\test.py", line 19, in covers
if topics.intersection(value):
AttributeError: 'str' object has no attribute 'intersection
But it works in the task. how do I run it?
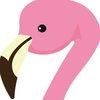
Dave StSomeWhere
19,870 PointsGood deal, more testing = more learning and just for fun, it is your code not mine hehe...
Notice that the error message is that a string doesn't have an intersection attribute.
You need to pass a set because sets have the intersection attribute. Notice that how I run the function is with the following statement - passing a set not a string:
print(str(covers({"Python"})))

Tor Ivan Boine
4,057 Pointsaha, that makes somewhat sense. Thanks. Wish these tasks (in general) were more informative. I don't have a photographic memory (if such a thing exists).