Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial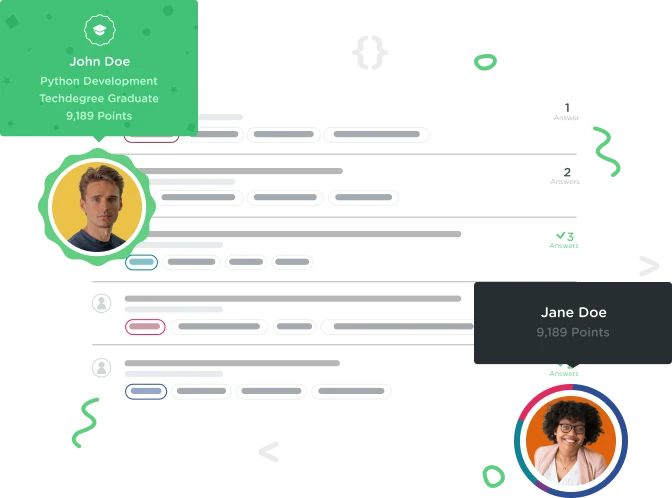

Michael McKinley
1,476 PointsCan someone help me with this code?
Pretty lost.
We need to add getter and setter methods to the fahrenheit property of the Temperature class. Add a getter method to the access the value of the fahrenheit property. Then add a setter method. When the user assigns a value to the fahrenheit property, the setter method will calculate and assign a value to the celsius property. (Note: Celsius = (Fahrenheit-32)/1.8))
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
fahrenheiht = newValue
celsius = (fahrenheit - 32)/1.8))
}
}
2 Answers

J.D. Sandifer
18,813 PointsExpanding a little on Kyle's great answer: You're never going to assign a value directly to a computed property. Remember, a computed property is just that, one that's computed based on other properties. It never has a stored value - it's simply re-calculated each time it's accessed. Setter methods change values on the other properties that the computed property references, thus changing what's returned when the computed value is accessed.
The challenge is an excellent example of why these properties are useful. We might want to talk about a temperature using either Celsius or Fahrenheit depending on who's using our app, but we only want to store one value. We can access the correct value and change that value using either temperature scale.
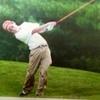
kjvswift93
13,515 PointsThe correct answer is this:
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
celsius = (newValue-32)/1.8
}
}
}
We only need to set the celsius variable it's equivalent fahrenheit value by using the conversion equation. No need to assign fahrenheit to it's own new value .
Bradan Jackson
20,558 PointsBradan Jackson
20,558 PointsI believe that should work, but you have an extra "h" in Fahrenheit, when you're setting Fahrenheit to the new value. Also, you have two close parentheses at the end of that statement that should not be there.
The code I used for the setter method was just
celsius = (newValue - 32)/1.8