Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial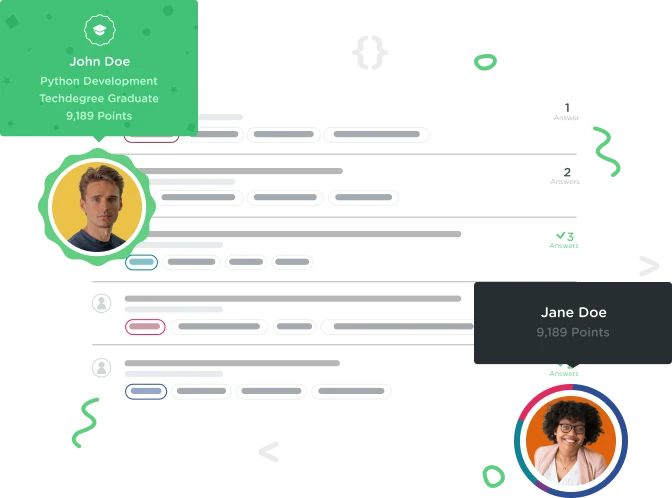

Vansh Agarwal
Courses Plus Student 3,965 PointsCan someone help me with this code challenge?
When I execute the code below, I get an error message in 'Preview', saying 'swift_lint.swift:351:54: error: value of type 'UIView' has no member 'layoutMarginsGuide' greenView.leftAnchor.constraint(equalTo: view.layoutMarginsGuide.leftAnchor, constant: 8),'. I don't know why this is happening as I tried doing this on XCode and it still worked. Please help me out. Thanks!
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
NSLayoutConstraint(item: blueView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .top, relatedBy: .equal, toItem: self.topLayoutGuide, attribute: .bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
NSLayoutConstraint.activate([
greenView.topAnchor.constraint(equalTo: blueView.bottomAnchor, constant: 8),
greenView.leftAnchor.constraint(equalTo: view.layoutMarginsGuide.leftAnchor, constant: 8),
greenView.rightAnchor.constraint(equalTo: view.layoutMarginsGuide.rightAnchor, constant: 8),
greenView.heightAnchor.constraint(equalToConstant: 75)
])
}
}
1 Answer
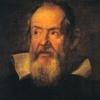
landonferrier
25,097 PointsVansh,
Here is the code that I got to pass the challenge:
class MyViewController: UIViewController {
let blueView = UIView()
let greenView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(greenView)
view.addSubview(blueView)
}
override func viewWillLayoutSubviews() {
super.viewWillLayoutSubviews()
blueView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.activate([
NSLayoutConstraint(item: blueView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 100.0),
NSLayoutConstraint(item: blueView, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 150.0),
NSLayoutConstraint(item: blueView, attribute: .left, relatedBy: .equal, toItem: view, attribute: .leftMargin, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .top, relatedBy: .equal, toItem: self.topLayoutGuide, attribute: .bottom, multiplier: 1.0, constant: 8.0),
NSLayoutConstraint(item: blueView, attribute: .right, relatedBy: .equal, toItem: view, attribute: .rightMargin, multiplier: 1.0, constant: -8.0)
])
// Add constraint code below
NSLayoutConstraint.activate([
greenView.bottomAnchor.constraint(equalTo: view.bottomAnchor, constant: 8),
greenView.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 8),
greenView.trailingAnchor.constraint(equalTo: view.trailingAnchor, constant: 8),
greenView.heightAnchor.constraint(equalToConstant: 75.0)
])
}
}
When activating the constraints, you don't need to use the layout guide to get the anchors. These can be accessed directly from the "view" property. If you have any questions, feel free to ask.