Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial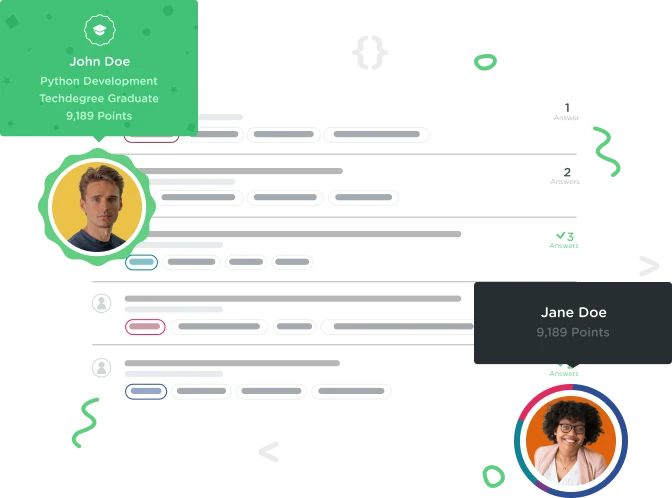

Andrei Oprescu
9,547 PointsCan someone help me with this confusing task?
Hello!
I am on this challenge that asks me a pretty confusing question:
Finish the prereqs function so that it recursively finds all of the prerequisite course titles in courses (like "Object-Oriented Python" is a prerequisite for "Django Basics"). You should add() the title of the prerequisite to the pres set and then call prereqs again with the child courses. In the end, return the prereqs set.
The code that I have been offered is at the bottom of this post.
Can someone please break the question down for me and help me a little?
Help would be greatly appreciated.
Also, can someone explain to me what set() does?
Thanks!
Andrei
from operators import add
courses = {'count': 2,
'title': 'Django Basics',
'prereqs': [{'count': 3,
'title': 'Object-Oriented Python',
'prereqs': [{'count': 1,
'title': 'Python Collections',
'prereqs': [{'count':0,
'title': 'Python Basics',
'prereqs': []}]},
{'count': 0,
'title': 'Python Basics',
'prereqs': []},
{'count': 0,
'title': 'Setting Up a Local Python Environment',
'prereqs': []}]},
{'count': 0,
'title': 'Flask Basics',
'prereqs': []}]}
def prereqs(data, pres=None):
pres = pres or set()
3 Answers
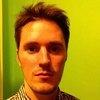
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsA set is a kind of data structure, here are the docs with a pretty good explanation. So set()
is creating a new instance of a set for you. In the code you've been given so far, there's a parameter pres
which might have a value or might be None
. Then in the first line of the function, pres = pres or set()
, we're setting the value of pres
to a new set object if there isn't one already, or we're using the existing set object that's passed in. This will be important later on.
The goal here is to have a set
object that contains all of the prerequisite courses. We're using a set as opposed to a list because it's possible some courses have the same prereq and we don't want to list them more than once.
Here's what we need to do now:
def prereqs(data, pres=None):
pres = pres or set()
# loop through each of the items in the `prereqs` property of the data
# for each prepreq, add it's title to the set
# then we want to add each of the prereq's prereqs to the set.
# ...This is where we'll use recursion.
# you'll want to call this function within itself
# ... the data argument will be the prereqs and the pres argument will be the existing set
Let me know if you need more help.

Andrei Oprescu
9,547 PointsHi again!
I have covered all of you advices and came up with this code:
def prereqs(data, pres=None):
pres = pres or set()
for prereq in data or courses:
pres.add(prereq['title'])
prereqs(prereq, pres=pres)
return pres
I still didn't get the challenge correct.
Can you tell me what I did wrong again?
Thanks
Andrei
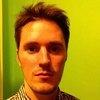
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThe problem is this line:
for prereq in data or courses:
We shouldn't be referencing the courses
object here inside the function, just reference data
. When the function is called, courses
will be passed in as an argument, and will become data
. Then when the function is called later recursively, data
will be assigned to the nested properties.
Also we need to loop through the prereqs
property on data
:
for prereq in data['prereqs']:

Andrei Oprescu
9,547 PointsThanks! I understand what i did wrong now.
Andrei
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsHi again!
I got the hang of what the question sais but I have a small problem...
When I write this code:
I get bummer: can't find prereqs.
Do you know why this might be?
Thanks!
Andrei
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 Points1) You don't want to access the
courses
object directly. That object is going to be passed in to thedata
parameter. The first time the function is called, it will becourses
, but when it call it recursively we'll be calling it on nested properties, so we can't be pointing at the top levelcourses
object every time the function is called.2) You need to return the set at the end of the function, otherwise none of the function calls will be useful, either the original function call or the recursive calls
3) You need to call the
add
method on the set, not anadd
function by itself. Give the method just one argument: the title.4) You need to access the keys with [] and string keys as opposed to dot notation. To be honest, I'm not sure why, but I've been immersed in JavaScript more than Python lately so I'm a bit out of practice.
5) When you recursively call
prereqs
, you're passing inprereq.prereqs
, but that's going too deep too soon. Remember thatprereq
is going to become thedata
parameter, and then inside the function you accessdata.prereqs
. So you just want to callprereqs(prereq, pres=pres)