Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial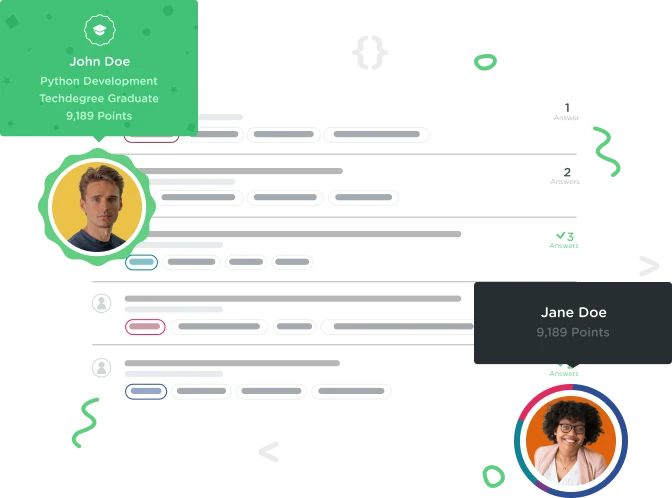

Alexander Gallardo
617 PointsCan someone help me with this damn inheritance problem??
I literally looked up how to do it and its still wrong is this a glitch?!?!?!
namespace Treehouse.CodeChallenges
{
class Polygon : Square
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
}
class Square: Polygon
{
public readonly int SideLength;
public Square (int sideLength): base (4)
{
SideLength = sideLength;
}
}
1 Answer

andren
28,558 PointsThere are two issues with your code:
The
Polygon
class should not inherit fromSquare
class.The
Square
class should be inside the same namespace as thePolygon
class.
Like this:
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square: Polygon
{
public readonly int SideLength;
public Square (int sideLength): base (4)
{
SideLength = sideLength;
}
}
}
Connor Whyte
1,395 PointsConnor Whyte
1,395 PointsWould someone be able to explain this to me? The base(4) specifically. I spent a while putting in a variety of variables like in the tutorial with x and y. I put in NumSides and numSides instead.
andren
28,558 Pointsandren
28,558 PointsThe
base
call is essentially you calling the constructor of the parent class. To put it a bit more simply, what would you write in order to create an instance ofPolygon
with 4 sides? You would typenew Polygon(4)
. The base call is essentially doing exactly that, it is calling thePolygon
constructor and passing in whatever value you provided in the base call.So the value you type in the base call is send directly to the parent's constructor method. That means that if the parent class' constructor had two parameter you would need to provide two arguments to the
base
call and so on.In the previous lecture the parent class' constructor required an
x
andy
argument, since those were also required by the constructor of the child class it was possible to just pass those parameters along, as passing in variables in the super call is valid. But that is not possible for the challenge code since the child constructor does not require you to pass in the number of sides, which is what thePolygon
constructor needs to be passed.Edit: Added a bit more info relating to the previous video lecture.