Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial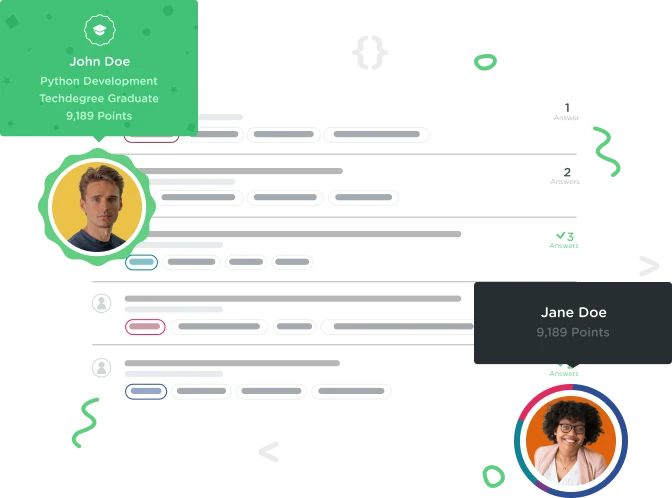

wizard xxdx
Python Development Techdegree Student 1,096 PointsCan someone help me with this python script?
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print("They are {} ticket remaning".format(tickets_remaining))
client_name = input("What is your name? ")
num_ticket = input("{}, How many ticket do you want? ".format(client_name))
num_ticket = int(num_ticket)
amount_due = num_ticket * TICKET_PRICE
print("The ticket due is ${}".format(amount_due))
user_info = input("Do you want to procced Y/N? ")
if user_info.lower() == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks any ways, {}".format(client_name))
print("Ticket soldout :( ")
4 Answers
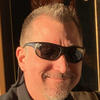
Peter Vann
36,429 PointsThis adds the handlers for trying to enter text instead of a number for the ticket quantity and if the user trys to buy more tickets than are available:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
if tickets_remaining > 1:
verb = "are"
remaining_s = "s"
else:
verb = "is"
remaining_s = ""
print("There {} {} ticket{} remaning (at $10.00 per ticket).".format(verb, tickets_remaining, remaining_s))
client_name = input("What is your name? ")
valid = False
while valid != True:
num_ticket = input("{}, how many tickets do you want to purchase? ".format(client_name))
try:
val = int(num_ticket)
except ValueError:
print("*** INVALID SELECTION ***")
continue
if val <= tickets_remaining:
valid = True
else:
print("*** ERROR ***: There {} only {} ticket{} remaining. Try again.".format(verb, tickets_remaining, remaining_s))
num_ticket = int(num_ticket)
if num_ticket > 1:
num_s = "s"
else:
num_s = ""
amount_due = num_ticket * TICKET_PRICE
print("The total amout due is ${}.00 for {} ticket{}.".format(amount_due, num_ticket, num_s))
user_info = input("Do you want to procced Y/N? ").lower()
if user_info == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks anyways, {}".format(client_name))
print("Tickets soldout! :( ")
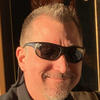
Peter Vann
36,429 PointsYo Wiz,
Here is my slightly augmented version of your code (so far):
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
if tickets_remaining > 1:
verb = "are"
remaining_s = "s"
else:
verb = "is"
remaining_s = ""
print("There {} {} ticket{} remaning (at $10.00 per ticket).".format(verb, tickets_remaining, remaining_s))
client_name = input("What is your name? ")
num_ticket = input("{}, how many tickets do you want to purchase? ".format(client_name))
num_ticket = int(num_ticket)
if num_ticket > 1:
num_s = "s"
else:
num_s = ""
amount_due = num_ticket * TICKET_PRICE
print("The total amout due is ${}.00 for {} ticket{}.".format(amount_due, num_ticket, num_s))
user_info = input("Do you want to procced Y/N? ").lower()
if user_info == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks anyways, {}".format(client_name))
print("Tickets soldout! :( ")
One thing it lacks is any error handling for cases where the user inputs unacceptable data (such as a number for the name, or a string for the ticket quantity) or if someone tries to buy more than the remaining tickets available).
I'll try to add some of that.
BTW, I do my testing of python code snippets here:
https://www.katacoda.com/courses/python/playground
I hope that helps.
Stay safe and happy coding!
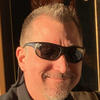
Peter Vann
36,429 PointsNow I've added a check to make sure the name is a string:
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
if tickets_remaining > 1:
verb = "are"
remaining_s = "s"
else:
verb = "is"
remaining_s = ""
print("There {} {} ticket{} remaning (at $10.00 per ticket).".format(verb, tickets_remaining, remaining_s))
valid = False
while valid != True:
client_name = input("What is your name? ")
try:
val = int(client_name)
except ValueError:
valid = True
else:
print("*** INVALID SELECTION ***")
valid = False
while valid != True:
num_ticket = input("{}, how many tickets do you want to purchase? ".format(client_name))
try:
val = int(num_ticket)
except ValueError:
print("*** INVALID SELECTION ***")
continue
if val <= tickets_remaining:
valid = True
else:
print("*** ERROR ***: There {} only {} ticket{} remaining. Try again.".format(verb, tickets_remaining, remaining_s))
num_ticket = int(num_ticket)
if num_ticket > 1:
num_s = "s"
else:
num_s = ""
amount_due = num_ticket * TICKET_PRICE
print("The total amout due is ${}.00 for {} ticket{}.".format(amount_due, num_ticket, num_s))
user_info = input("Do you want to procced Y/N? ").lower()
if user_info == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks anyways, {}".format(client_name))
print("Tickets soldout! :( ")
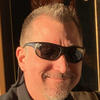
Peter Vann
36,429 PointsIn this (should be the last) version, I added a handler for the case if the user just hits enter instead of actually typing something for the name.
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
if tickets_remaining > 1:
verb = "are"
remaining_s = "s"
else:
verb = "is"
remaining_s = ""
print("There {} {} ticket{} remaning (at $10.00 per ticket).".format(verb, tickets_remaining, remaining_s))
valid = False
while valid != True:
client_name = input("What is your name? ")
if (len(client_name) == 0):
print("*** INVALID SELECTION ***")
else:
try:
val = int(client_name)
except ValueError:
valid = True
else:
print("*** INVALID SELECTION ***")
valid = False
while valid != True:
num_ticket = input("{}, how many tickets do you want to purchase? ".format(client_name))
try:
val = int(num_ticket)
except ValueError:
print("*** INVALID SELECTION ***")
continue
if val <= tickets_remaining:
valid = True
else:
print("*** ERROR ***: There {} only {} ticket{} remaining. Try again.".format(verb, tickets_remaining, remaining_s))
num_ticket = int(num_ticket)
if num_ticket > 1:
num_s = "s"
else:
num_s = ""
amount_due = num_ticket * TICKET_PRICE
print("The total amout due is ${}.00 for {} ticket{}.".format(amount_due, num_ticket, num_s))
user_info = input("Do you want to procced Y/N? ").lower()
if user_info == "y":
print("SOLD!!!")
tickets_remaining -= num_ticket
else :
print("Thanks anyways, {}".format(client_name))
print("Tickets soldout! :( ")
Probably the next step (and I'll leave it to you, unless you have more questions) would be to refactor the code to break-up some of the functionality into functions, so the main execution is more readable.
I hope that helps.
Stay safe and happy coding!

wizard xxdx
Python Development Techdegree Student 1,096 PointsThanks for your assistance, I've understood the problem.
boi
14,242 Pointsboi
14,242 PointsYou need help with what exactly?