Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial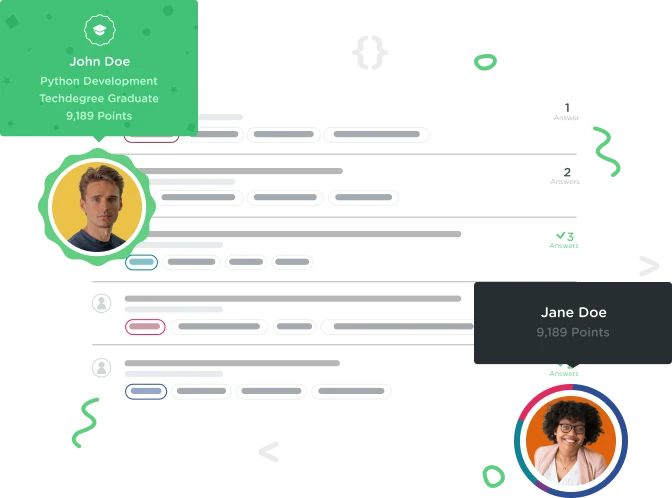

mryoung
4,156 PointsCan someone help walk me through reversing this loop?
Hello, I have a slider I'm building with a next and previous button.
I've already made the next button functional:
It loops through the slides array, with an index counting the slides, when the slides reach the end it'll loop back around. As this loop is going on, the images displayed disappear on each click and a new one appears.
var slideIndex = 1;
next.addEventListener('click', function () {
for(let i = 0; i < slides.length; i++){
slides[i].style.display = 'none';
}
slideIndex++;
if(slideIndex > slides.length){
slideIndex = 1;
}
slides[slideIndex - 1].style.display = 'block';
})
But I'm confused how to REVERSE this for the previous button being clicked. I've tried and tried, and could only get it to jump back to the last image. and then it stops.
I get this error: Uncaught TypeError: Cannot read property 'style' of undefined at HTMLElement.<anonymous> (apps.js:41)
prev.addEventListener('click', function () {
for(let i = slides.length -1; 0 <= i; i--){
slides[i -1].style.display = 'none';
}
slideIndex++;
})
Can you walk me through the logic needed to complete this?
2 Answers
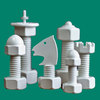
Steven Parker
229,732 PointsThe offset indexes and reverse looping make things a bit confusing. It might be simpler to first determine which slide is about to become the active one, and then both handlers can use the same code to update the display:
var slideIndex = 0;
const updateSlides = index => {
slides.forEach(slide => {
slide.style.display = "none";
});
slides[index].style.display = "block";
};
next.addEventListener("click", e => {
if (++slideIndex > slides.length) {
slideIndex = 0;
}
updateSlides(slideIndex);
});
prev.addEventListener("click", e => {
if (--slideIndex < 0) {
slideIndex = slides.length - 1;
}
updateSlides(slideIndex);
});

mryoung
4,156 PointsThanks 🙂
mryoung
4,156 Pointsmryoung
4,156 PointsI’ve made another post on here with my solution. I used a few resources and pieced together the answer I needed.
Basically I used a counter index, if statements to check if the loop is at the beginning or end and to reset them accordingly), and then passed a 1 or -1 to the function to add into the index.
I couldn’t get Index++ or Index- - to work like that, so it was just easier to pass it into a function like that.
Thanks for your help 🙂
Steven Parker
229,732 PointsSteven Parker
229,732 PointsI didn't have your context to check it, but I expected that code to be ready-to-run.
I hoped it helped some in any case, and happy coding!