Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial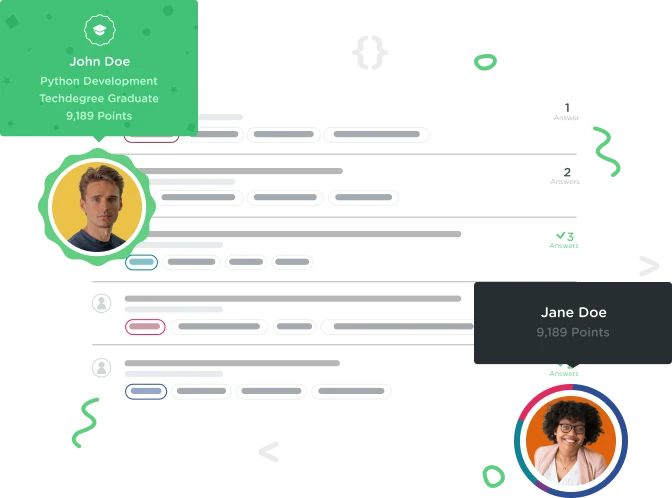
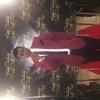
Billy Potts
Courses Plus Student 2,344 Pointscan someone help with the method
Given the struct below in the editor, we want to add a method that returns the person’s full name. Declare a method named getFullName() that returns a string containing the person’s full name. Note: Make sure to allow for a space between the first and last name
struct Person {
let firstName: String
let lastName: String
func getFullName(firstName: String, lastName: String) -> String {
var getFullName: ["Person"] = []
return getFullName
}
}
2 Answers

Keli'i Martin
8,227 PointsOK, I see the issue. Because this is an instance method of the struct, you don't need to have parameters in the function. It will simply use the values already present in the struct. The full struct should look like this:
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
let fullName = "\(firstName) \(lastName)"
return fullName
}
}

Keli'i Martin
8,227 PointsSo the biggest problem with your function is that you are trying to return an array of String, but the function definition says you should be returning a String. Definitely a big problem and probably causing your code to not compile at all.
Your function just needs to concatenate the two String parameters that are being passed in and return the resulting String. Try this:
func getFullName(firstName: String, lastName: String) -> String {
let fullName = "\(firstName) \(lastName)"
return fullName
}
Notice that we are adding a space between the first and last name, as requested.
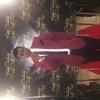
Billy Potts
Courses Plus Student 2,344 Pointsi tried it and there is no compile error but it just won't approve it so i can move on

Keli'i Martin
8,227 PointsLemme try going through the exercise and see what's going on. The exercises can be very particular about what you are trying to input and output.