Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial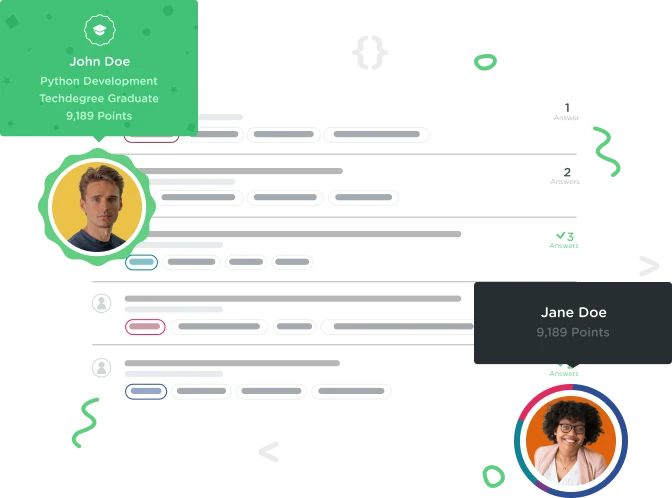
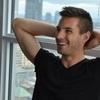
evanpavan
5,025 PointsCan someone lend a hand with the code challenge?
I'm getting a compiler error below. How do I introduce a variable in the loop without this error or avoid using a variable altogether?
"FrogStats.cs(11,20): error CS0103: The name `runningTotalTonguelength' does not exist in the current context Compilation failed: 1 error(s), 0 warnings"
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
foreach(Frog frog in frogs)
{
double runningTotalTonguelength = runningTotalTonguelength + frog.TongueLength;
}
return runningTotalTonguelength / frogs.Length;
}
}
}
3 Answers
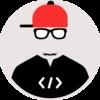
Luis Duenas
5,967 PointsIf you declare a variable outside of a static method, and you want to use it inside of the method, the variable has to be static too.
You can learn more about static classes and static class members variables here.
This code worked for me in the challenge:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double runningTotalTonguelength = 0.0;
foreach(Frog frog in frogs)
{
runningTotalTonguelength = runningTotalTonguelength + frog.TongueLength;
}
return runningTotalTonguelength / frogs.Length;
}
}
}
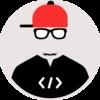
Luis Duenas
5,967 PointsYou need to declare your variable outside of the scope of the foreach loop, remember that a variable only lives in the scope where it was created.
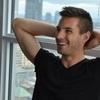
evanpavan
5,025 PointsThanks. This is the code that ended up working.
Can anyone tell me why the runningTotalTonguelength variable had to be a static variable? The definition of static methods and classes makes sense but static member variables are not clear to me.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double runningTotalTonguelength = 0;
public static double GetAverageTongueLength(Frog[] frogs)
{
foreach(Frog frog in frogs)
{
double runningTotalTonguelength = runningTotalTonguelength + frog.TongueLength;
}
return runningTotalTonguelength / frogs.Length;
}
}
}