Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial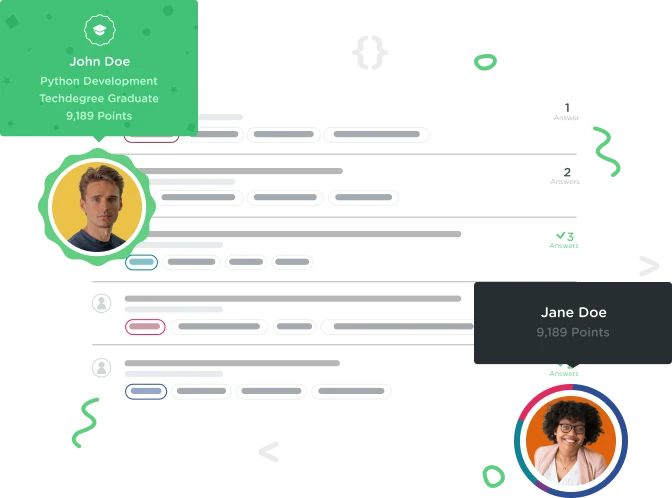

markmneimneh
14,132 Pointscan someone please elaborate on what this challenge is asking for?
This challenge is ambiguous at best:
I tried this, which returns date time:
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
Remember, you can't set "years" on a timedelta!
Consider a year to be 365 days.
Example
time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(an_int, a_string): a_begin_string = "datetime(" an_end_string = ")" a_separator = ", "
a_return=""
if a_string.lower() == 'minutes':
new_time = starter + datetime.timedelta(minutes=an_int)
a_return = str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute)
return datetime.datetime.strptime(a_return,'%Y, %m, %d, %H, %M')
elif a_string.lower() == 'hours':
new_time = starter + datetime.timedelta(hours=an_int)
a_return = str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute)
return datetime.datetime.strptime(a_return,'%Y, %m, %d, %H, %M')
elif a_string.lower() == 'years':
new_time = starter + datetime.timedelta(days=an_int*365)
a_return = str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute)
return datetime.datetime.strptime(a_return,'%Y, %m, %d, %H, %M')
I got a bummer
I then tried this, which returns exact string as requested in the challenge ... e.g.
time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34);
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
def time_machine(an_int, a_string): a_begin_string = "datetime(" an_end_string = ")" a_separator = ", "
a_return=""
if a_string.lower() == 'minutes':
new_time = starter + datetime.timedelta(minutes=an_int)
a_return = a_begin_string + str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute) + an_end_string
return a_return
elif a_string.lower() == 'hours':
new_time = starter + datetime.timedelta(hours=an_int)
a_return = a_begin_string + str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute) + an_end_string
return a_return
elif a_string.lower() == 'years':
new_time = starter + datetime.timedelta(days=an_int*365)
a_return = a_begin_string + str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute) + an_end_string
return a_return:
I still got a bummer
What exactly is being asked?
Thank you for your help / clarifications
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(an_int, a_string):
a_begin_string = "datetime("
an_end_string = ")"
a_separator = ", "
a_return=""
if a_string.lower() == 'minutes':
new_time = starter + datetime.timedelta(minutes=an_int)
a_return = str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute)
return datetime.datetime.strptime(a_return,'%Y, %m, %d, %H, %M')
elif a_string.lower() == 'hours':
new_time = starter + datetime.timedelta(hours=an_int)
a_return = str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute)
return datetime.datetime.strptime(a_return,'%Y, %m, %d, %H, %M')
elif a_string.lower() == 'years':
new_time = starter + datetime.timedelta(days=an_int*365)
a_return = str(new_time.year) + a_separator
a_return += str(new_time.month) + a_separator
a_return += str(new_time.day) + a_separator
a_return += str(new_time.hour) + a_separator
a_return += str(new_time.minute)
return datetime.datetime.strptime(a_return,'%Y, %m, %d, %H, %M')
1 Answer
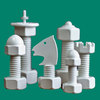
Steven Parker
229,744 Points
You didn't implement all of the required cases.
While your solution is perhaps a good deal more complicated than it needs to be, the concept is essentially sound. But the challenge asks that your function handle "minutes", "hours", "days", or "years"; and your implementation handles only 3 of these four cases (all but "days"). If you add additional code to handle that case, you should pass.
I would, however, suggest that you take the opportunity to re-consider your approach and see if you can find a way to perform the required task that would require only one or two lines for each case.
markmneimneh
14,132 Pointsmarkmneimneh
14,132 PointsHello
thanks for pointing out the missing days. It passed after adding another elif for days.
The complexity came about because of the bummer thing ... I did not realize that I was missing days and thought I am expected to return a string like ... datetime(2015, 10, 21, 16, 34);
in any case, this is the simple code
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29) def time_machine(an_int, a_string): if a_string.lower() == 'minutes': return starter + datetime.timedelta(minutes=an_int) elif a_string.lower() == 'hours': return starter + datetime.timedelta(hours=an_int) elif a_string.lower() == 'days': return starter + datetime.timedelta(days=an_int) elif a_string.lower() == 'years': return starter + datetime.timedelta(days=an_int * 365)
Thank you very much for the catch