Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial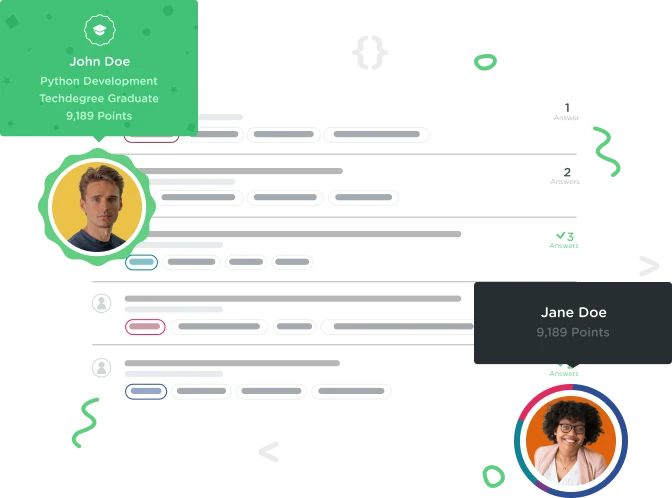
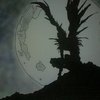
Lucas Santos
19,315 PointsCan someone please explain "Binding"
I have a really good grasp on javascript and understood the course pretty well but one thing I really do not understand is "Binding".
Example of a function he used in this course to bind elements and functions.
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
Why is he making an event out of an element and setting it to another function. He doesn't even call the function with the parenthesis he just calls the name and sets it to that event handler. Please explain why he did that and what are the benefits of binding or what is it?
Also couldn't he just call the function on the click instead of having to "bind it"?
1 Answer

Chris Shaw
26,676 PointsHi Lucas,
Binding is the process of assigning a reference to a function by assigning a function name to the value assignment of an object/event, this is pretty much the simplest explanation I can give without confusing you even more.
The reason it's called binding is as explained we're assigning a reference to the function deleteTask
for example, on the surface we can't see this reference because it happens behind the scenes in the heart of every browsers JavaScript engine, one thing we don't see is a physical memory pointer is being created but that's not important to know since the browser manages that for us.
If we were to simple call deleteTask()
when assigning it to the onclick
event for example the function would execute straight away without assigning the function reference to onclick
which would result in broken code as anytime we would click on the element nothing would happen.
Hope that helps explains why you don't need to use the parentheses and what binding is at a basic level.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI'm really trying to get this but still have some difficulties. Can you tell me:
What do you mean exactly when you say "reference"
Why bind an element with a function? How does that help us?
I understand the concept of binding like you explained on top its assigning the function name to the value of the an element with an even handler but do not really understand how this benefits us. What's its purpose?
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsAs I said you don't need to worry too much about this keyword as in JavaScript an reference can be substituted with binding, explaining the core details about references will end up confusing you ten times more as it involves C/C++.
I think you're confusing what's actually happening in the code provided and what you think is happening, for example take the following line.
editButton.onclick = editTask;
This code is calling the
onclick
event for theeditButton
element, events in JavaScript working by binding either an explicit function name and/or an anonymous function to the event type which is the same whether we're using theonclick
property or theaddEventListener
method.In both instances the
editTask
becomes a physical reference foronclick
which results in theeditTask
function being called every time the user clicks on the element, as mentioned we can also use an anonymous function like the below example shows.There are no benefits to binding functions to event handlers aside from they are called whenever the event is triggered by the user, the simple purpose is to allow code to be executed whenever user interaction occurs, without these functions we wouldn't be able to execute our code.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI see ok thanks! I think I got it.