Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial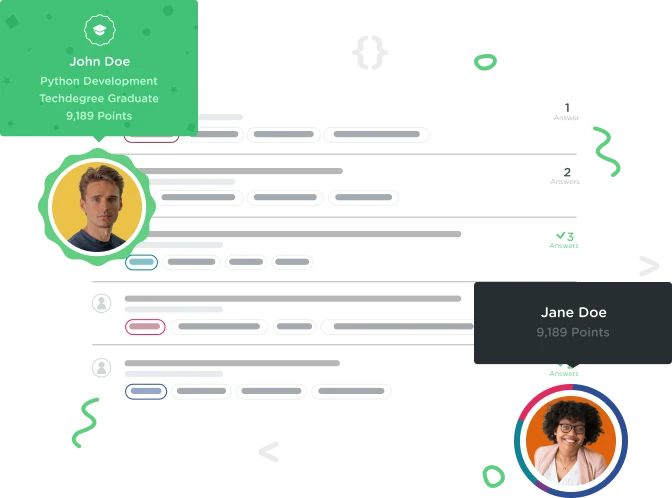
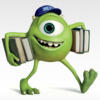
Tobias Edwards
14,458 PointsCan someone please explain 'enumerate' to me, please?
I have come across so many definitions of 'enumerate', but I still don't understand.
Could someone please explain 'enumerate' to me in "Layman's" terms please. This is definitely the hardest part of Python for me to understand thus far.
I would appreciate examples, and when to use it.
Thank you :)
2 Answers
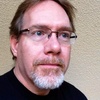
Chris Freeman
Treehouse Moderator 68,457 PointsTo loop over all the items in a list you can simply use the format:
for item in my_list:
# do something with item
But what if you wanted to also utilize the index of an item, you might use:
for idx in range(len(my_list)):
# print each item with it's index
print("{0}. {1}".format(idx, my_list[idx]))
enumerate()
provides a way to simplify code for this very common case.
for idx, item in enumerate(my_list):
# print each item with it's index
print("{0}. {1}".format(idx, item))
So for each item in the iterable (such as my_list
, or characters in a string, etc), enumerate
return a tuple of the o-based count and an item. In the example above, this tuple is unpacked into the variables idx
and item
.
enumerate
-like behavior could be done using:
for idx, item in zip(range(len(my_list)), my_list):
# same as using enumerate but MUCH less readable

rdaniels
27,258 PointsThe enumerate function lets you iterate over the index-value pairs, where the indices are supplied automatically. eg: enumerate(iterable) will iterate over (index, item) pairs, for all items in iterable.... source: Beginning Python
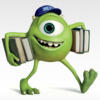
Tobias Edwards
14,458 PointsThanks but I've read that before. Could you give examples and uses, please?
Tobias Edwards
14,458 PointsTobias Edwards
14,458 PointsWow! Thank you sooooo much :)
But, just a small thing: when you format variables in using
print("{0}. {1}".format(idx, my_list[idx]))
Why is it you use a '0' and a '1' in the curly braces? I'm only ever used to seeing empty curly braces, sorry
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsChanging from empty
{}
to numbered{0}
specifies the order to apply the positional arguments given toformat()
. In the code above, adding the numbers doesn't change anything. Just my preferred style.One application would be if you needed to use an argument twice:
print("{0} {0} {1}!".format("hip", "hooray"))
To get
hip hip hooray!
Expanding lists as format argument doesn't alway arrive in the desired order. Given the list:
lst = ['Tobias', 'Edwards']
How could I print "last_name, first_name"? Let's use numbered format fields:
print("{1}, {0}".format(*lst))
Yeilds
Edwards, Tobias
Tobias Edwards
14,458 PointsTobias Edwards
14,458 PointsChris, you the man! Thank you so much!