Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial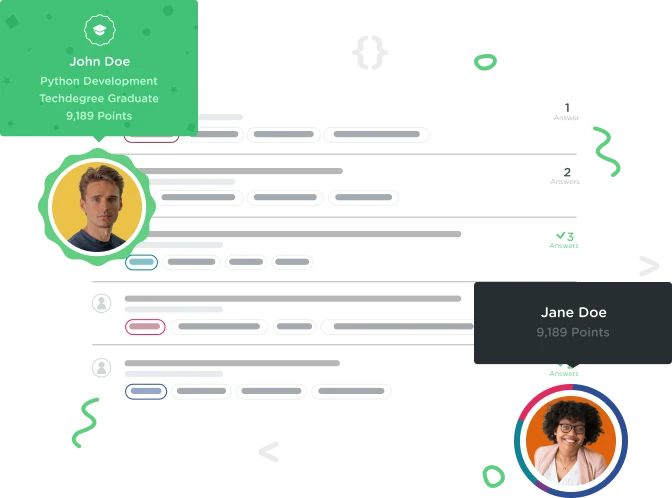

Matt George
2,507 PointsCan someone please explain the core concepts that were taught in this video more simply?
This was a very difficult tutorial, struct initialization. Up until now, I understand everything that's taught. Please please help me understand the deal with merging the arrays and initializing stuff. I don't want to fall behind.
Thank you!!
3 Answers
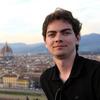
Eduardo Scodino
5,083 PointsFirst, remember that you have to get the values from other Model file (MusicLibrary.swift). So you start the struct creating the stored properties that will hold the values:
var title: String?
var description: String?
var icon: UIImage?
var largeIcon: UIImage?
var artists: [String] = []
var background: UIColor = UIColor.clearColor()
Second, to get the values, you have to "import" the dictionary (remember there is an dictionary inside that array) and define which array you will use to initialize your properties:
let musicLibrary = MusicLibrary().library // import the entire array to this constant
let playlistDictionary = musicLibrary[index] //selecting the dictionary that is inside the array
Once you have that defined, you now just have to retrieve the attributes that is inside the dictionary to the properties:
title = playlistDictionary["title"] as! String!
description = playlistDictionary["description"] as! String!
let iconName = playlistDictionary["icon"] as! String!
icon = UIImage (named: iconName)
let largeIconName = playlistDictionary["largeIcon"] as! String!
largeIcon = UIImage (named: largeIconName)
artists += playlistDictionary["artists"] as! [String]
Since its a dictionary (and a dictionary can store any kind of object), you have to define for each property which kind of object is. That's why the use of the syntax "as! String!", "as! [String]" etc.
I hope it can help a bit.
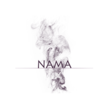
NOUR A ALGHAMDI
Courses Plus Student 5,607 Pointsthank you Mr. Pasan Premaratne also you Eduardo Scodino i got a clear vision for this tutorial . . . perfect society :)
إحسان :) means: goodness
Nour .

Sercan Lir
Courses Plus Student 2,153 PointsThank you Eduardo. I have a follow-up question: Wouldn't it be easier to call different dictionary items by creating an instance? For example: "let library1 = MusicLibrary().library[0] library1["title"]" Gives me the title of the first item in the dictionary. I feel that this method is way easier for the purpose of this small scale application. But in a case, where we have larger data, the method used by the teacher might be more applicable. Do you agree?
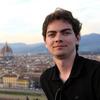
Eduardo Scodino
5,083 PointsDefinitely it makes sense. And that is a good question. I tried the solution that you proposed and it worked perfectly. I just have to add " ! " to unwrap the optional, but this is a minor point.
Unfortunately I don't have enough knowledge to answer your question, but I will follow this topic just in case someone else help with some information.
Maybe Pasan Premaratne @pasanpr can help.
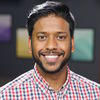
Pasan Premaratne
Treehouse TeacherHi Sercan Lir,
That snippet of code will work, but its a long term problem. You never know how big your applications will grow and from the very beginning you want to structure your code in such a way that it is flexible, reusable, and bug free.
The analogy here (even if this example is silly) is if you are building a one story home that one day you might want to expand to a two or three story home. Your approach is akin to saying let's build a foundation for a one story home, and then in the future if we want to expand, we'll lift out the entire house and change the foundations to make it a two story home. Further down if you want it to be a three story, you lift the house out again...
The approach can work, but it will lead to long term headaches. You will build an entire app to rely on that dictionary, since you are calling it explicitly by creating an instance. What if the dictionary changes or we end up using data from the internet (as we do in subsequent courses)? Then you will have to go back and change everything related to the model.
Instead, by letting the model know only the minimal amount it needs to know about the data source, you're creating a more reusable solution. In our case, all the model knows, is the key to use for the value it's looking for. It doesn't matter where that key is stored.
Hope that makes sense.
Short Version: Write code for long term use and you will be a better developer.