Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial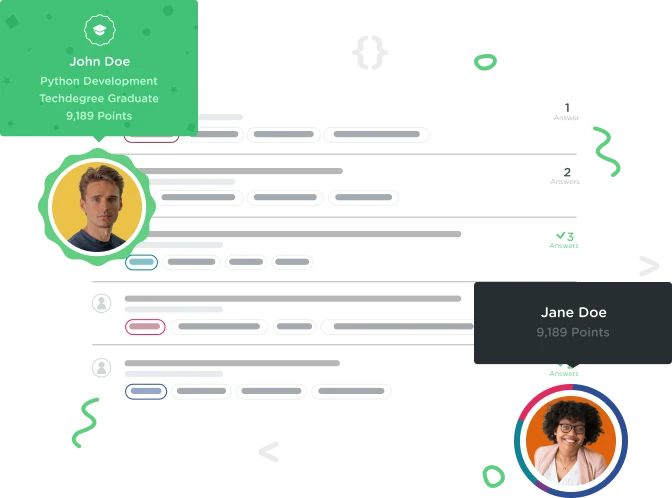
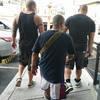
Omar G.
3,620 PointsCan someone please explain the Exception flow quiz to me?
print("A") try: result = "test" + 5 print("B") except ValueError: print("C") except TypeError: print("D") else: print("E") print("F")
I got the answer correct but I guessed. I don't understand exactly what is happening here for both questions on the quiz. Can anyone help?
2 Answers
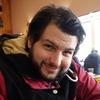
Eric M
11,545 PointsI've added some comments to the code block that hopefully help to explain
print("A") # prints the character 'A'
try: # opens a try block
result = "test" + 5 # adds a string to an int, this throws a TypeError
print("B") # gets skipped, we go straight to the except block
except ValueError: # we didn't get a ValueError (we got a TypeError)
print("C") # so this gets skipped too
except TypeError: # we arrive here right after result = string + int
print("D") # prints the character 'D'
else: # the else block only executes if no exceptions occured
print("E") # we had a TypeError so this is skipped
print("F") # prints the character 'F' (not part of the try block)
Usually, when something goes wrong Python raises an exception - an error that describes what went wrong. When a try block is opened, if any statements within it raise an exception (like a ValueError, TypeError, KeyError, etc) then Python checks to see if there's an except block that matches that exception type, if there is it executes that block instead of doing what it normally would (it would normally terminate your program and print the error).
Sometimes we might want python to continue even if there's an error, or we might want a very specific way of handling that error. For instance, say we're looking up an item in a dictionary, we get the dictionary key from the user and return the value associated with that key, but if the user enters a key that's not in the dictionary we'll get a key error. Crashing here isn't very user friendly, so instead we use a try/except Key Error block to let the user know the value couldn't be found (and maybe giving them another go) instead of the program terminating.

Richard Li
9,751 Pointsprint("A") try: result = "test" + 5 print("B") except ValueError: print("C") except TypeError: print("D") else: print("E") print("F")
the flow are: print A first, then try to add test to 5 and print B if the adding met no problem.
if any ValueError(like test is not giving) during the adding, it will not carry out the print('B') part and directly print C;
if any TypeError(like test is not able to add to 5) during the adding, then it will not carry out the print('B') part and print D;
if no exception has been trigger( the adding is successful) it will print B and go to 'else:' part to print E.
And Finally Print F which has no relation to the whole try area just like print('A') at the beginning.
So the answer should the adding be successful is A-B-E-F.
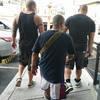
Omar G.
3,620 PointsThank-you for taking the time to help me.
Omar G.
3,620 PointsOmar G.
3,620 PointsThank-you. Adding the comments really helped me see what was happening. I will use this to review the lesson. I really appreciate it.