Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial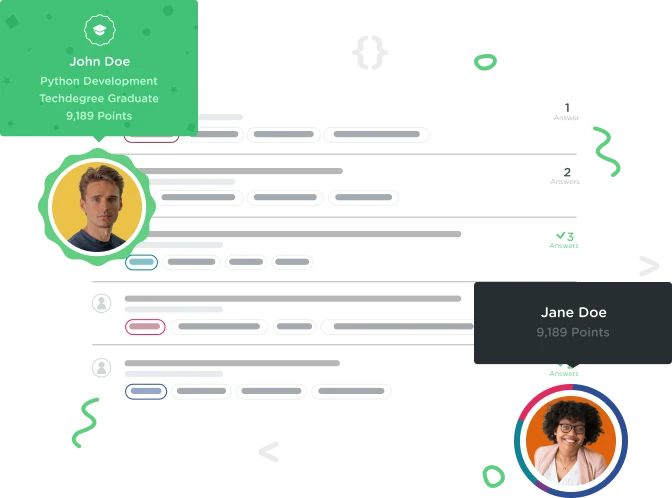
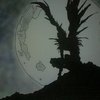
Lucas Santos
19,315 PointsCan someone please explain this code
Im stuck on this quiz and I believe it's asking me what this code would output on the screen.
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$output = $number . $output;
}
}
echo $output;
?>
3 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsWhen this particular piece of code starts executing, there are four variables initialized at the top. First, you can ignore $sum
because it's not used elsewhere in the code. $i
is 0
, $total
is 4
because there are 4 elements in the $numbers
array, and $output
is an empty string.
On the first iteration of foreach
, $i
gets immediately increased to 1
. Then we check if it's smaller that $total
. Because it is, the current number from the $numbers
array (in this case it's the first element — 1
) gets concatenated with the $output
and the resulting string is assigned as the new value of the $output
variable, which now equals to "1"
.
On the second iteration, $i
is increased to 2
. It's still smaller than $total
, so now the second element of the array (2
) is concatenated with $output
("1"
) and the resulting string ("21"
) is assigned as the new value of $output
.
The same is true for the third iteration, only now the resulting string is "321"
.
In the fourth iteration, $i
is now 4
and as it's not smaller than $total
, no value re-assignment of $output
happens.
So, the final output is the resulting string from the third iteration: 321
.

Guillaume Maka
Courses Plus Student 10,224 Points<?php
// declare an array of 4 numbers
$numbers = array(1,2,3,4);
// assign the length of $number array to $total -> 4
$total = count($numbers);
// declare a variable $sum and initialize it to 0
$sum = 0;
// declare a variable $output and initialize it to an empty string
$output = "";
// declare a variable $i and initialize it to 0
$i = 0;
// do a foreach loop to grab each element in the array
foreach($numbers as $number) {
// track how many element we have already take
// ex: at the first iteration, $i = 0 + 1
$i = $i + 1;
// check we don't exceed the total sze of the array
// ex: at the first iteration, $i = 1 , $total = 4, so 1 < 4 then enter into the if statement
if ($i < $total) {
// concatenate the given $number to the $output
// ex: at the first iteration, $number = 1, $ouptut = ''
$output = $number . $output;
// $output = '1'
}
}
// print the output to the screen
// $output = '321'
echo $output;
?>
Hope that will help you.
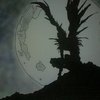
Lucas Santos
19,315 PointsThanks a lot this helps!
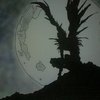
Lucas Santos
19,315 PointsThanks a lot this helps!
Lucas Santos
19,315 PointsLucas Santos
19,315 Pointscrystal clear
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsHey so when ever you concatenate a number or value to an empty variable it will always add it to the left meaning the beginning of the empty string rite? I would think it would be like reading and it adds the numbers to the rite like 123 instead of 321 but I guess not.
Guillaume Maka
Courses Plus Student 10,224 PointsGuillaume Maka
Courses Plus Student 10,224 PointsTo get 123 you just need to do the opposite
$output = $output . $number;
That was your question ?
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsI see ok got it thanks!