Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial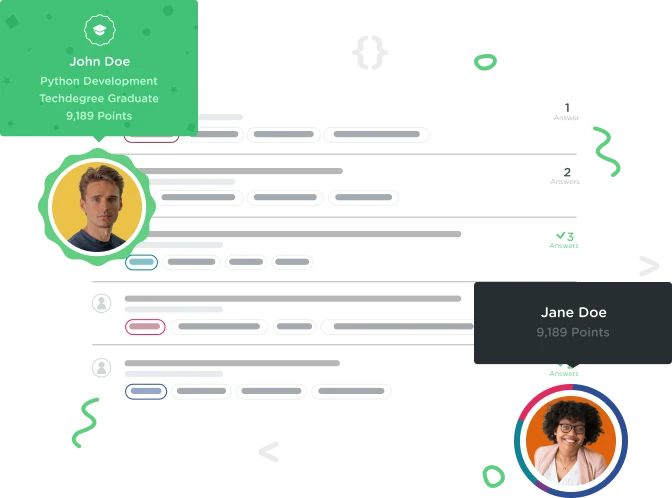

Waylan Sands
Courses Plus Student 5,824 PointsCan someone please explain this code
This was the answer to a code challenge. We have gone over stucts, initializers, and optionals though I thought this was a little behond the scope of the lessons so far. I only found the answer by Googling and am still lost.
What's happening here? What's the use of this struct?
struct Book { let title: String let author: String let price: String? let pubDate: String?
init?(dict: [String: String]) {
guard let title = dict["title"], let author = dict["author"] else {
return nil
}
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
}
2 Answers
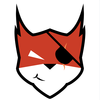
Rickey Paape
24,122 PointsStructs are a type of framework to build objects in Swift.
Think of a struct, and classes for that matter, as a category. The Book struct defines a book as having an author, title, price, pubDate. This could be used in a book store's database, we can even add a var quantity to the struct so we can track how many are in the store.
For example, we have 2 books (I'll skip initialization):
let bookOne = Book()
let bookTwo = Book()
Now the store has sold 2 copies of bookOne and received 27 of bookTwo in a shipment. We could adjust the quantities based on these numbers:
bookOne.quantity -= 2 \\ 2 books sold
bookTwo.quantity += 27 \\ 27 copies received
They are both books, they have the same property categories (price, quantity, title, etc.), so rather than create the framework everytime you add a book, the Book struct allows you to build the framework once and use it across multiple versions of books. Hope this helps

Waylan Sands
Courses Plus Student 5,824 PointsThanks for the response. I appreciate you explaining the use of structs but what I'm looking for was a specific example of how to use this struct which was the answer to a lesson within the iOS course.
struct Book { let title: String let author: String let price: String? let pubDate: String?
init?(dict: [String: String]) {
guard let title = dict["title"], let author = dict["author"] else {
return nil
}
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
} }