Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial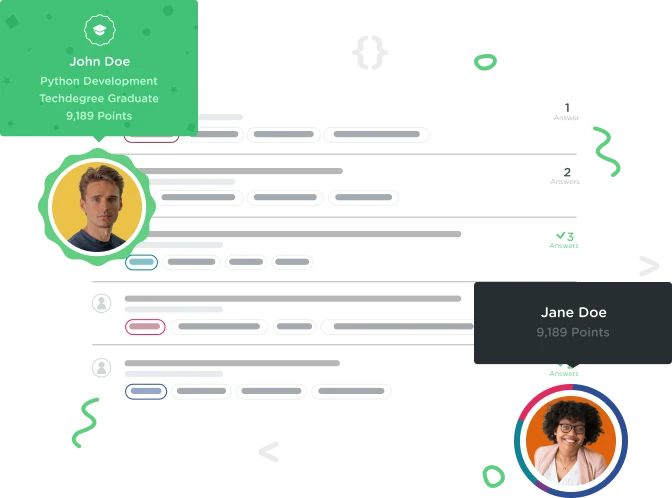

Wilson Majok Kuol Bol
1,431 PointsCan someone please explain to me this problem?
Check if each student has a GPA of 4.0. If the student has a GPA of 4.0, use the student's name variable to display "NAME made the Honor Roll". If not, use the variable to display "NAME has a GPA of GPA".
I can understand this description!
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if($studentOneGPA == 4.0) {
echo '$studentOneName made the Honor Roll';
}
else {
echo '$studentOneName has a $studentOne of $studentTwoGPA';
}
?>
4 Answers
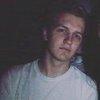
Anton Williams
5,157 PointsHi,
I think first of all you need to check both students, not just one, this could be done with two if-statements as this is a simple example, but if you needed to do it for more in the future you'd want to change it to a function. But for this example I wouldn't worry about that.
I'm not sure if the challenge wants to check if the GPA is at least 4.0 or exactly 4.0, but it seems more logical to check if it is 4.0 or higher which is what I've done below.
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if($studentOneGPA >= 4.0) {
echo '$studentOneName made the Honor Roll';
}
else {
echo '$studentOneName has a GPA of $studentOneGPA';
}
if($studentTwoGPA >= 4.0) {
echo '$studentTwoName made the Honor Roll';
}
else {
echo '$studentTwoName has a GPA of $studentTwoGPA';
}
?>
Here is an explanation to the question. You need to test both student's GPAs to see if they make 4.0, if they do make 4.0 you echo "StudentName made the Honor Roll", if they do not make 4.0 then you echo "StudentName has a GPA of StudentGPA".

Wilson Majok Kuol Bol
1,431 PointsThanks for answer but it doesn't work still.
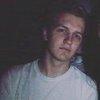
Anton Williams
5,157 PointsAh it's because the quote marks. When you use ' marks the text in between is completely plain text.
'this is plain text'
where as this will echo the variable
"this shows a $variable"

Victor Utama
6,934 PointsYeah, i'm having the same issue.

Victor Utama
6,934 PointsStrangely... it's the formatting.
<?php $studentOneName = 'Dave'; $studentOneGPA = 3.8;
$studentTwoName = 'Treasure'; $studentTwoGPA = 4.0;
//Place your code below this comment if ($studentOneGPA === 4.0) { echo $studentOneName . " made the Honor Roll"; } else { echo $studentOneName . " has a GPA of " . $studentOneGPA; } if ($studentTwoGPA === 4.0) { echo $studentTwoName . " made the Honor Roll"; } else { echo $studentTwoName . " has a GPA of " . $studentTwoGPA; } ?>
Got that from the other thread. I did something similar and it works for me.

Jason Wist
2,483 PointsThey want you to use string concatenation.