Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial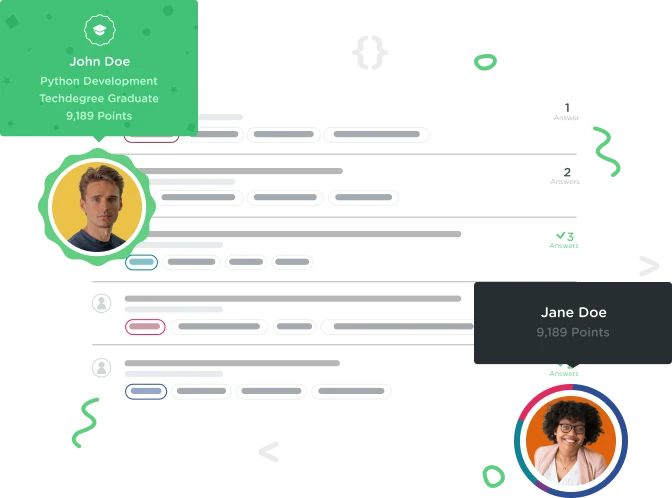

Chris Rubio
1,643 PointsCan someone please give me a different example of casting without using treet.?
the whole object obj = treet; is so confusing , can someone please try to give me a different example with different variable names .
1 Answer
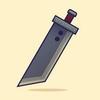
Allan Clark
10,810 PointsEssentially this is talking about the practical use of inheritance/object hierarchy. He is using Object because in Java everything (thats not a primitive type) is a Object at its root.
For example, suppose we have an application for tracking automobiles. We could have a Car class, a Truck class, and a Motorcycle class. They will all have characteristics in common so we can have them all inherit from an Automobile class.
What this video is showing you is how the types of variables behave when dealing with an object hierarchy like this. In this example any Car object can be held in a variable of type Car or of type Automobile, but the variables and methods that are specific to the Car class can't be accessed if the variable is Automobile type and would require a down cast.
Car mazda = new Car();
mazda.getTrunkSpace(); //this will work because the variable is a car
Automobile auto = mazda;
auto.getTrunkSpace(); //this will error because not all automobiles have trunks
Car sameCar = (Car) auto;
sameCar.getTrunkSpace(); //works again bc we downcast
excuse my rusty java syntax lol. hope this helps
Chris Rubio
1,643 PointsChris Rubio
1,643 PointsThis helps, Thank you :)
Allan Clark
10,810 PointsAllan Clark
10,810 PointsNo problem, let me know if there is anything else with this i can help clear up. If all is good please select as 'Best Answer' to mark this post as closed.
Chris Rubio
1,643 PointsChris Rubio
1,643 PointsI do have another question , regarding the object Arrays, can you explain what is he doing there , and explain instanceOF ?
Allan Clark
10,810 PointsAllan Clark
10,810 PointsFor sure! So in some cases we may need to have an Array of objects of different types. Since everything in Java has Object as its root class we can just declare the type of the Array to be Object and then we can store any object type in any index of that array. But with that flexibility comes a draw back, because we can store any type in any index, if we just pull one of the indexes out of the array we don't know what its type is. Thats where instanceOf comes in, that will check if the object is of a certain type.
Back to the Automobile example; suppose we have a method to add up the number of tires needed for an Array of Automobiles
We could generalize it even more by having the parameter be Object[] but then we would definitely need to add in explicit instanceOf checks for Car and Truck since we would no longer be guaranteed to have only Automobiles in the array.
David Smith
10,577 PointsDavid Smith
10,577 PointsThis answer was perfect, made a light blub click on when I read it.