Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial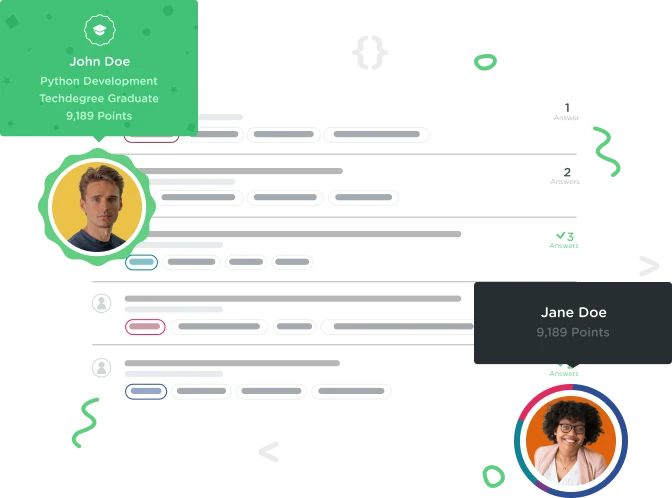
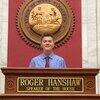
Ronald Greer
Front End Web Development Techdegree Graduate 56,430 Pointscan someone please help
it says i have a syntax error
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public int compareTo(Object obj){
if(obj instanceof BlogPost){
BlogPost bp = (BlogPost) obj;
return 0;
}
int dateComp = mCreationDate.compareTo(other.mCreatonDate);
return 1;
}
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
3 Answers

Yanuar Prakoso
15,196 PointsHi Ronald
As the challenge task says: You need to implements Comparable interface to the class and then add Object type into method called compareTo then you need to modify the class name first:
import java.util.Objects;
public class BlogPost implements Comparable{
do not forget to add import java.util.Objects since you want to add an Object later in the compareTo method.
Then you were asked to produce a compareTo method that accepts an object as a parameter. Have it always return 1 for this first step so please just do that first:
@Override
public int compareTo(Object object) {
return 1;
}
Later on this challenge you will get the opportunity to use more complicated code in the compareTo method. For this first task just keep it simple okay.
I hope that can help
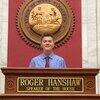
Ronald Greer
Front End Web Development Techdegree Graduate 56,430 PointsStill not working

Yanuar Prakoso
15,196 PointsThis is my code for passing the first challenge:
package com.example;
import java.util.Date;
import java.util.Objects;//<--- import this
public class BlogPost implements Comparable{//<-- do not forget to implements Comparable
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
//erase all your added code from before
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public int compareTo(Object object){//<-- add this code
return 1;
}
}
please check again
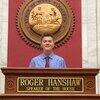
Ronald Greer
Front End Web Development Techdegree Graduate 56,430 Pointsohhhhhh your showing task one i am on task 3. could you show me task3?

Yanuar Prakoso
15,196 Pointsjust modify your compareTo method to be:
public int compareTo(Object object) {
BlogPost blogPost = (BlogPost)object;
if (equals(blogPost)) {
return 0;
}
return mCreationDate.compareTo(blogPost.mCreationDate);
}