Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial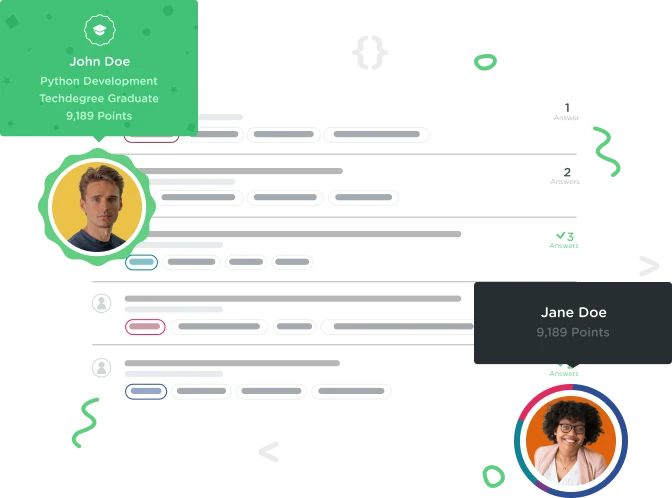
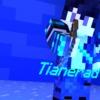
Tianerad 999
4,063 PointsCan someone please help me figure out what's wrong?
This is what I have to do: You probably already spotted the error in the drive method that accepts laps. You can drive way more laps than our battery can handle. Let's fix it.
Add logic to the drive method so that it throws an IllegalArgumentException if there aren't enough bars to support the laps request. Remember it takes 1 bar of energy to go around a lap.
Please try to help me out with this! I await anyone's response!
My code so far:
class GoKart { public static final int MAX_BARS = 8; private String color; private int barCount; private int lapsDriven;
public GoKart(String color) { this.color = color; }
public String getColor() { return color; } public void charge() { barCount = MAX_BARS; }
public boolean isBatteryEmpty() { return barCount == 0; }
public boolean isFullyCharged() { return MAX_BARS == barCount; }
public void drive() { drive(1); }
public void drive(int laps) { int totalLaps = lapsDriven += laps; if (totalLaps > MAX_BARS) { throw new IllegalArgumentException("You cannot drive this much!"); } barCount = laps; } }
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
int totalLaps = lapsDriven += laps;
if (totalLaps > MAX_BARS) {
throw new IllegalArgumentException("You cannot drive this much!");
}
barCount = laps;
}
}
2 Answers

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHello Tianerad 999
Thanks for sharing your code.
All you need to do is to make sure the ride is not available when there is less than 1 bar count using if-statement then throw the Exception.
Please see how I did it on the below code snippet inside drive() method.
public void drive(int laps) {
lapsDriven += laps;
if (barCount<1){
throw new IllegalArgumentException("You cannot drive this much!");
}
barCount -= laps;
}
Cheers Man, keep going..
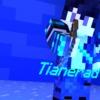
Tianerad 999
4,063 PointsOh! Ok, thanks for making things clearer!