Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial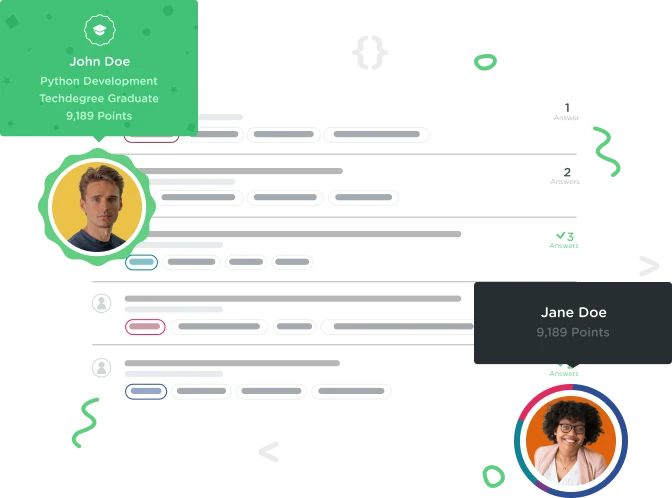

Ilya Sikharulidze
Courses Plus Student 1,073 PointsCan someone please help me, I don't understand how to use 'return' value in this case
using System;
class Program
{
static string CheckSpeed(double speed)
{
if(speed > 65.0)
{
Console.WriteLine("too fast");
}
else if(speed < 45.0)
{
Console.WriteLine("too slow");
}
else
{
Console.WriteLine("speed OK");
}// YOUR CODE HERE
}
static void Main(string[] args)
{
// This should print "too slow".
Console.WriteLine(CheckSpeed(44));
// This should print "too fast".
Console.WriteLine(CheckSpeed(88));
// This should print "speed OK".
Console.WriteLine(CheckSpeed(55));
}
}
5 Answers

Ilya Sikharulidze
Courses Plus Student 1,073 PointsThanks! It works, now I understand!
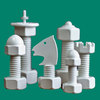
Steven Parker
231,269 PointsYou're pretty close there. But instead of outputting the strings directly (using "Console.WriteLine
"), your code should return the string values (using "return
").

Ilya Sikharulidze
Courses Plus Student 1,073 PointsThanks, I understand that, but I don't understand how to exactly to implement the "return"
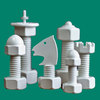
Steven Parker
231,269 PointsWhere you currently have the term "Console.WriteLine
" (in 3 places), replace it with the word "return
". Optionally, you can also remove the parentheses around the string (replacing the open parenthesis with a space).
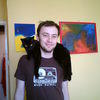
Michal Lysiak
9,651 PointsProgram.cs(6,19): error CS0161: 'Program.CheckSpeed(double)': not all code paths return a value [/workdir/workspace.csproj] Program.cs(27,9): error CS0127: Since 'Program.Main(string[])' returns void, a return keyword must not be followed by an object expression [/workdir/workspace.csproj] Program.cs(29,9): error CS0127: Since 'Program.Main(string[])' returns void, a return keyword must not be followed by an object expression [/workdir/workspace.csproj] Program.cs(31,9): error CS0127: Since 'Program.Main(string[])' returns void, a return keyword must not be followed by an object expression [/workdir/workspace.csproj] Program.cs(29,9): warning CS0162: Unreachable code detected [/workdir/workspace.csproj]
The build failed. Please fix the build errors and run again.
I've done as you advise and still not working
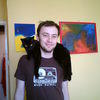
Michal Lysiak
9,651 Pointsusing System;
class Program {
static string CheckSpeed(double speed)
{
if(speed > 65.0)
{
Console.WriteLine("too fast");
}
else if(speed < 45.0)
{
Console.WriteLine("too slow");
}
else
{
Console.WriteLine("speed OK");
}// YOUR CODE HERE
}
static void Main(string[] args)
{
// This should print "too slow".
return(CheckSpeed(44));
// This should print "too fast".
return(CheckSpeed(88));
// This should print "speed OK".
return(CheckSpeed(55));
}
}
what's wrong?
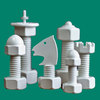
Steven Parker
231,269 PointsInstead of posting a question as an "answer" to another one, always start a fresh new question and show your code there.
But here's a hint: all your code should go in the "CheckSpeed" routine, the "Main" should remain as it was supplied.
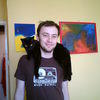
Michal Lysiak
9,651 PointsYeah, but it still doesn't work and shows me an error as above :(
Steven Parker
231,269 PointsSteven Parker
231,269 PointsIlya Sikharulidze — Did you mean to mark your own comment as "best answer"?
(You can still change it)