Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial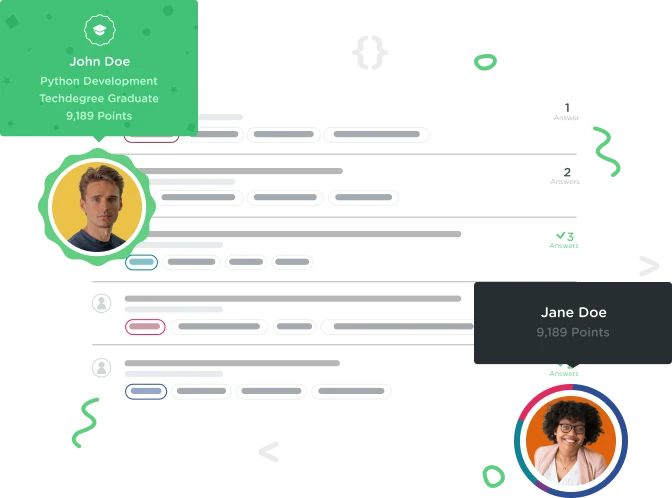
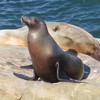
otarie
984 PointsCan someone please help me with my code? Thanks!
we're supposed to create a function combo() that takes two lists and returns a list of tuples that contain items from both lists with the same index. for ex: (list_1[0], list_2[0]), (list_1[1], list_2[1]), etc...
thanks for helping
# combo(['swallow', 'snake', 'parrot'], 'abc')
# Output:
# [('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
# If you use list.append(), you'll want to pass it a tuple of new values.
# Using enumerate() here can save you a variable or two.def combo(thingone, thingtwo):
def combo(thingone, thingtwo):
n = 0
while n < len(thingone):
return (thingone[n], thingtwo[n])
n=+1
2 Answers

Chase Marchione
155,055 PointsHi otarie,
You want:
- To return a list of tuples.
Here's a way to do it:
- Declare an empty list of tuples (see the line below the function declaration header in my code example), which will have values appended to it as a result of the iterations of your loop.
- Use a loop that runs for as many indecies as one of your tuples has (this works because we can assume each iterable is the same length, so it doesn't matter which one we choose.) Your use of the len method is good, but the loop structure needs tinkering.
- Append to the previously declared list of tuples for each iteration, so that you may return a list of tuples that has the new values you need passed in. (Also, we want to make the return statement run after all the iterations are ran.)
Example:
def combo(thingone, thingtwo):
tuples = []
for index in range(len(thingone)):
tuples.append((thingone[index], thingtwo[index]))
return tuples
Hope this helps.
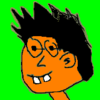
michaelangelo owildeberry
18,172 Pointsdef combo(thingone, thingtwo):
n = []
for index, item2 in enumerate(thingtwo):
n.append( (thingone[index], item2) )
return n