Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial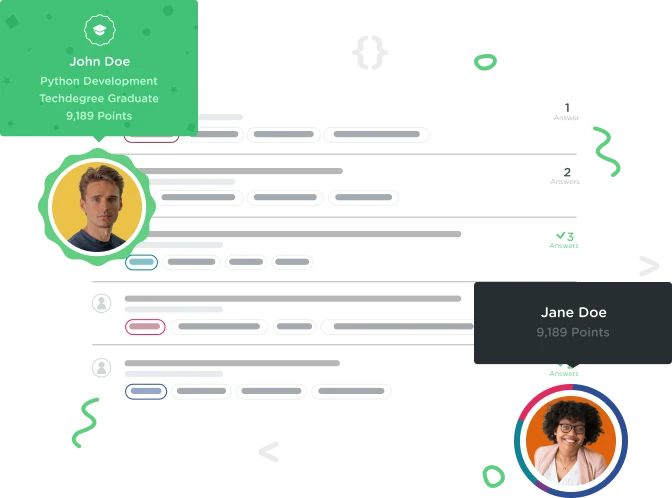

Ethan Tam
2,684 PointsCan someone please tell me what I did wrong in this code challenge. I am really confused about methods.
I watched the video on methods multiple times but something is still not clicking. I certain questions but I don't know the material well enough to put into words.
struct Person {
let firstName: String
let lastName: String
func fullName (Name: Person) -> String {
return firstName + " " + lastName
}
}
2 Answers
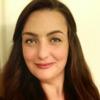
Jennifer Nordell
Treehouse TeacherHi there, Ethan! I received your request for assistance and have worked to try and put together an answer for you. However, I'm not sure I understand exactly what you're not understanding (if that makes any sense). You are wondering why you shouldn't send in a Person, and I'm wondering why you would. I've thought about it a bit and the only scenario I can come up with to need to send in one instance of a Person to another would be if the method needs to act based on a separate instance of the object. So I've expanded upon this example to see where this might be applicable (if a little far-fetched). This might also clear up some understanding about "self" and what it means.
Here is my example:
struct Person {
let firstName: String
let lastName: String
func fullName () -> String {
return firstName + " " + lastName // returns the full name of the current instance of the person
}
func greetOtherPerson(other: Person) {
let greeting = "Hi there, \(other.firstName)! My name is \(self.fullName())." //Use the other person's name and give your own full name
print(greeting) // print the greeting
}
}
let me = Person(firstName: "Jennifer", lastName: "Nordell") // create an instance of Person with my name
let you = Person(firstName: "Ethan", lastName: "Tam") // create an instance of Person with your name
me.greetOtherPerson(other: you)
//Output: Hi there, Ethan! My name is Jennifer Nordell.
you.greetOtherPerson(other: me)
//Output: Hi there, Jennifer! My name is Ethan Tam.
This is, of course, buildable and runnable in a Playground
Other than giving this expanded example, I'm not sure what else I can add, but let me know if you need more clarification!

Ethan Tam
2,684 PointsThank you so much for your insight. I guess the most confusing part is that I don't understand how a function can produce something when it doesn't take in anything. How can a function have no parameter?
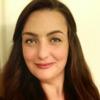
Jennifer Nordell
Treehouse TeacherNot every function needs outside information. A good portion of them do, yes, but you can make a function that simply displays something.
function sayHi() {
print("Hello Ethan! Pleased to meet you!")
}
sayHi()
But the problem with these functions (generally speaking) are that they do something very specific and very limited as they generally do something very specific with either something hard-coded or something available in the global scope.
Hope this helps!
edited for addition information
In the code in this challenge a struct is used. Structs come with their own default initializer which means that the firstName
and lastName
constants are automatically accessible to the function without them being passed in.
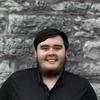
Michael Hulet
47,913 PointsTo clarify a bit, in the case of this question, the fullName
function is a method. All methods are functions, but not all functions are methods. If the function is declared on a class
, struct
, or enum
, it's a method, but if it stands all by itself, it's just a function. Methods are all given one parameter, even if you declare none. That parameter is self
inside the method. When you compile the code to pass this challenge, it's actually turned into a low-level C call that makes your method actually look something like this:
func fullName(self: Person) -> String{
return self.firstName + " " + self.lastName
}
iOS will eventually call your method like this:
var current: Person // Assume this variable is equal to the current Person instance that's being operated on
fullName(self: current)
A good concrete example of how this is implemented is how Python methods work. In Python, you have to write the self
keyword for the method to work. This is the above class
, but written in Python:
class Person:
def __init__(self, first_name, last_name):
self.first_name = first_name
self.last_name = last_name
def full_name(self):
return self.first_name + " " + self.last_name
See how Python makes you write out the self
keyword every time? It works exactly the same in Swift under the hood, but Swift doesn't make you write self
every time
Ethan Tam
2,684 PointsEthan Tam
2,684 PointsI got the answer and completed the code challenge, but how come my code is wrong when I put Person as a parameter?