Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial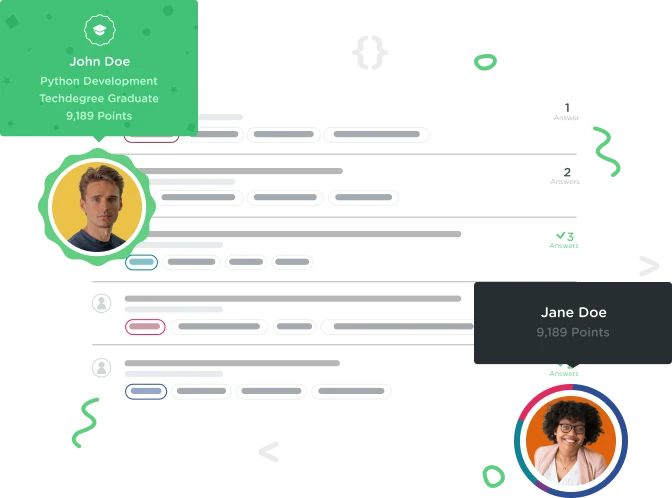

Jan M
1,053 PointsCan someone please walk me through this question? I have no Idea what to do even once presented with the answer :/
I am talking about this question:
"Make a function named add_list that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input()."
I watched all the Videos till now and felt 'quite' confident, however I noticed that I always struggled with the Code Challenges.. are they supposed to be this hard or was I just not paying attention?
Could someone please explain this question and the answer to me?
Thanks alot
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
4 Answers
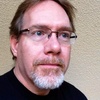
Chris Freeman
Treehouse Moderator 68,457 PointsLet's walk through it. Given the problem:
Make a function named add_list that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input().
Step 1. Make a function named add_list that takes a list.
This means "[Define] a function named add_list
that takes a list
[as an argument].". A simple function can be defined as below. Note the argument can be any name you wish. It will be assigned the list
when the function is called. It is typical to choose a name that best represents what the variable will contain. pass
is a keyword and is used as a placeholder for a block of code. pass
does nothing.
def add_list(lst):
pass
Step 2. The function should then add all of the items in the list together....
Adding all of the items in the list will require a loop. (there are other ways but for beginning coders learning about loops is important). Replacing pass with our loop:
def add_list(lst):
# loop over every item in lst
for item in lst:
# add this item to the running total of items
total = total + item
The above code is not complete. The issue is total
doesn't have a initial value. This will cause an error the first time through the loop. Fix by adding initialization to total
:
def add_list(lst):
# initialize total
total = 0
# loop over every item in lst
for item in lst:
# add this item to the running total of items
total = total + item
Step 3. ... and return the total.
Including a return
statement is crucial. without the return
the function's result is set to None
by default.
def add_list(lst):
# initialize total
total = 0
# loop over every item in lst
for item in lst:
# add this item to the running total of items
total = total + item
# return the total
return total
Note that he return
statement indentation is the same as the for
loop statement. A common beginner mistake is indenting the return
too much which makes it part of the for
loop code block. This would cause the function to return after the first pass through the loop.
Chris Grazioli
31,225 PointsI think I'm having issues with the naming conventions and trying to pass in the argument "list" into the function the problem asks us to create
def add_list(list)
for num in list:
list_total+=num
return list_total
I see that list gets highlighted red in Workspaces so its probably a keyword or something, but when I try to pass something else like a_list I seem to be running into the same sort of issue
Chris Grazioli
31,225 Pointssince I figured out what I was missing, I have a new question, why aren't my forum posts being formatted... I wrapped the code in question with the "```" (triple back-ticks) and specified python, how is the MOD getting the cool formatting??? Backticks are the little guys under the tilde key right?
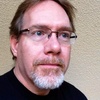
Chris Freeman
Treehouse Moderator 68,457 PointsThere are two requirements for cool formatting:
Triple backtick: ```python
A blank link before and after the code block.
I added a blank line to your comment.
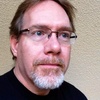
Chris Freeman
Treehouse Moderator 68,457 PointsThe root issue is list_total
is not initialized to 0. The statement
list_total += num
is equivalent to list_total = list_total + num
.
The first time this executes the right-side of the assignment has no value for list_total
. Solve by adding
list_total = 0
before the for
loop.
Chris Grazioli
31,225 PointsI seriously can't believe I'm screwing this up because of a missing colon " : "
Chris Grazioli
31,225 PointsMohamed THIAM The item which should be named something else is the argument you are passing into the function. It can be called anything from "a_list_of_numbers" to "xyz" that part doesn't matter. You are telling the function def what the function will accept. In this case it's a list of numbers which the function will loop through and add to a local variable called list_total, it must be initialized to zero to start, or it will have no value.
Jan M
1,053 PointsJan M
1,053 PointsThanks a lot for the quick and detailed reply, it really helped me understand this stuff!
Mohamed THIAM
1,091 PointsMohamed THIAM
1,091 PointsHi Chris,
Thank you. This is super helpful. I'm still confused though as to:
Cheers,
Mohamed.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsHi Mohamed,
Where "item" comes from and what is its nature
item
comes from thefor
statement. In this statement,for
takes each element of the iterable (in this case, the passed inlst
) one at a time and assigns it to the local variableitem
(this can be any variable name) then executes the code block following thefor
statement. So the loop will run for each of the elements in the iterablelst
. For exampleRange returns an iterable
(0, 1, 2, 3, 4)
. Thefor
loop will run 5 times, with number assigned to the next element in the iterable.When can I know that I need to assign a starting value to "total" for the operation to run? Is it a default procedure anytime I want to +/-* something to another item? I don't understand the logic here..
In any statement, the variables must be defined before they are referenced. When using a
+=
or-=
, these are the equivalent of having the results variable (in this casetotal
on both sides of the equation. So on the first loop, when the right-side of the statement is evaluated,total
will not yet have a value. There are two ways this can be resolved:So, yes, every time you use a
+=
,-=
, etc. you will need to initialize the variable somewhere above that statement. This is also true if you use the long form:total = total + item
Mohamed THIAM
1,091 PointsMohamed THIAM
1,091 PointsChris, I though I had it figured out but then this happened. Can you tell me the difference between these two pieces? Both of them work but the logic sort of escapes me.
If I understand correctly, in "total = 3", 3 refers to the 3 list items. But those were never defined. Or was it an implicit variable of the challenge?
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsMohamed,
The second solution is passing because of guessing the correct answer the challenge is looking for. In each loop,
total
is reset to 3, then incremented by the value ofitem
. At the end of the loop the total will always be "3 + last item in list".This trivial code will also pass:
Mohamed THIAM
1,091 PointsMohamed THIAM
1,091 PointsThanks for being so helpful Chris! This is super awesome.