Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial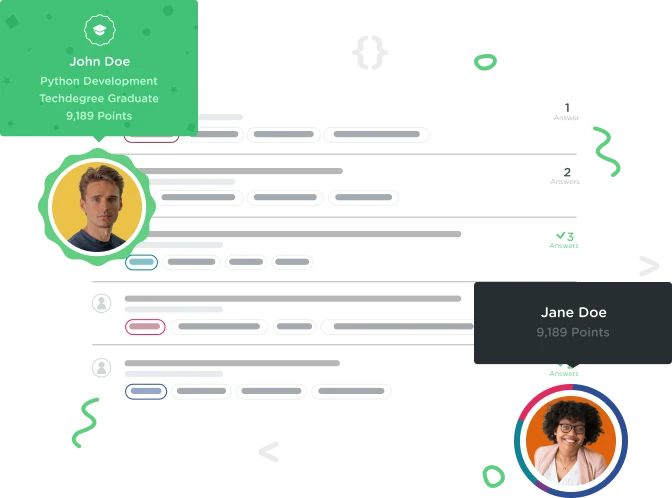

Peter Huang
5,427 PointsCan someone provide the answers for this challenge?
what am I missing for my code to get this to work?
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times10 = [];
// times10 should be: [10,20,30,40,50,60,70,80,90,100]
// Write your code below
numbers.forEach(total => {
if( let i = 0; i < numbers.length; i++ ) {
times10 *= 10;
}
});
1 Answer
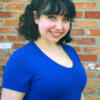
Bella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsHi Peter!
I'm happy to help guide you to a passing answer!
First things first, for each already iterates through the array. You don't need to also add an for
loop to access each number. Let's start by taking your multiplication out of the for
loop:
numbers.forEach(total => {
times10 *= 10;
});
From here we need to evaluate what should be multiplied by 10. You currently have it set to times10
, the empty array that will hold our results. We need it instead to be the values from the numbers
array. You've already defined each number in the original array with the function total
. We could keep it like this, but it's kind of a deceiving name. Let's instead go ahead and change both the function name and multiplication variable to number
instead:
numbers.forEach(number => {
number *= 10;
});
Now, let's have another look at the question. It wants us to multiply each number and then add it to the end of the times10
array. To do this we need to use the push() method. Let's add the start of this method:
numbers.forEach(number => {
number *= 10;
times10.push();
});
The last step is to define what should be pushed to the times10
array, in this case it's the number
value. Adding this we get our final, passing answer:
numbers.forEach(number => {
number *= 10;
times10.push(number);
});
Happy coding!
Peter Huang
5,427 PointsPeter Huang
5,427 PointsThank you so much. I really appreciate your time in explaining.
Bella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsBella Bradbury
Front End Web Development Techdegree Graduate 32,790 PointsAbsolutely! Glad I could help :)