Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial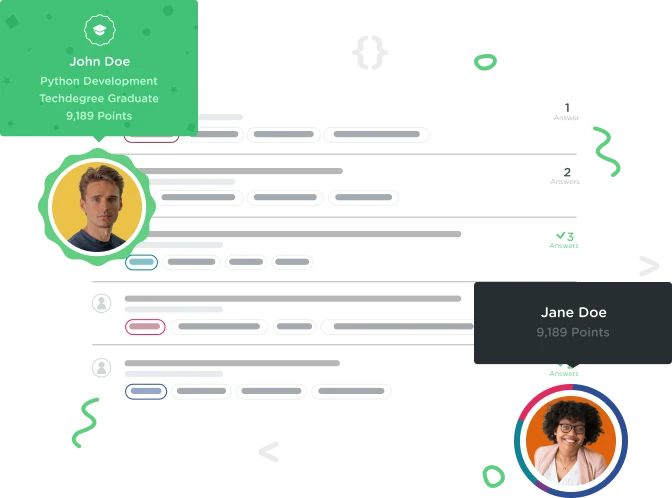

Tristan Gaebler
6,204 PointsCan someone review my code..
I'm getting an indentation error on my final game.py script. I can't seem to spot it. Can anyone help?
import sys
from character import Character
from monster import Dragon
from monster import Troll
from monster import Goblin
class Game:
def setUp(self):
self.player = Character()
self.monsters = [
Goblin(),
Troll(),
Dragon()
]
self.monster = self.getNextMonster()
def getNextMonster(self):
try:
return self.monsters.pop(0)
except IndexError:
return None
def monsterTurn(self):
if self.monster.attack():
print("{} is attacking..".format(self.monster))
if input("Dodge? y/n").lower() == 'y':
if self.player.dodge:
print("You dodged the attack")
else:
print("You got hit anyway...")
self.player.hitpoints -= 1
else:
print("{} hit you for one point".format(self.monster))
self.player.hitpoints -= 1
else:
print("{} isn't attacking this turn".format(self.monster))
def playerTurn(self):
playerChoice = input("Attack: Rest: quit").lower()
if playerChoice == 'a':
print("You're attacking {}!".format(self.monster))
if self.player.attack():
if self.monster.dodge():
print("{} dodged your attack".format(self.monster))
else:
if self.player.levelUp:
self.monster.hitpoints -= 2
else:
self.monster.hitpoints -= 1
print("You hit {} with your {}".format(self.monster, self.player.weapon))
else:
print("You missed")
elif playerChoice = 'r':
self.player.rest()
elif playerChoice = 'q'
sys.exit()
else:
self.playerTurn()
def cleanUp(self):
if self.monster.hitpoints >= 0:
self.player.experince += self.monster.experince
print("You killed {}".format(self.monster))
self.monster = getNextMonster()
def __init__(self):
self.setUp()
while self.player.hitpoints and (self.monster or self.monsters):
print("\n"+"="*20)
print(self.player)
self.monsterTurn()
print("-"*20)
self.playerTurn()
self.cleanUp()
print("\n"+"="*20)
if self.player.hitpoints:
print('You Win')
elif self.monster or self.monsters:
print('You Lose')
else:
sys.exit()
Game()
3 Answers

Trevor Currie
9,289 PointsRun this on your code:

Tom Holland
24,288 Pointselif playerChoice = 'q' is missing the colon at the end

zhulin peng
4,472 Pointsi only spotted a small mistake, but it should still let you run the code tho:
if input("Dodge? y/n").lower() == 'y': if self.player.dodge:
it should be if self.player.dodge():