Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial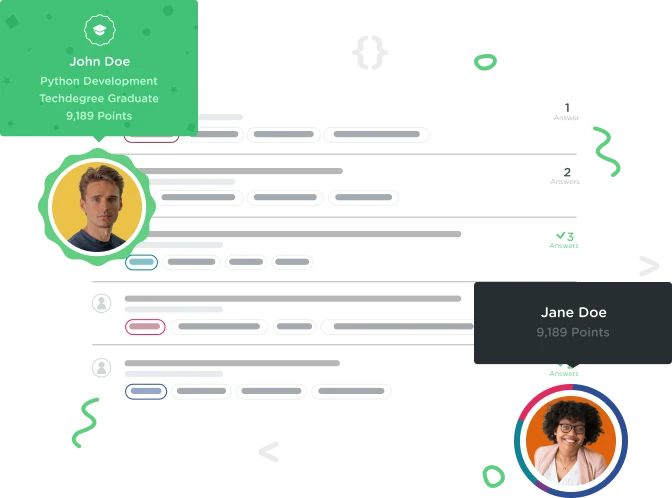

walter fernandez
4,992 PointsCan someone teach me what each line does?
this code is correct, I fond it on internet, I just want to understand please someone.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string = string.lower()
word_dict = {}
word_list = string.split( )
for sel_word in word_list:
count = 0
for word in word_list:
if sel_word == word:
count +=1
word_dict[sel_word] = count
return word_dict
3 Answers

Robin Goyal
4,582 Pointsdef word_count(string):
string = string.lower() # Creates a lowercase version of the string
word_dict = {} # Creates a dictionary for the frequency of the words in the string
word_list = string.split( ) # Splits the string into a list of all of the words
# i.e "This is a sample string" -> ["This", "is", "a", "sample", "string"]
for sel_word in word_list: # Selects each word in the list
count = 0 # Initializes a counter for each select word
for word in word_list: # Loops a second time through each word in the word list
if sel_word == word: # Checks the number of times the select word appears in the list
count +=1 # and updates the counter
word_dict[sel_word] = count # Updates the dictionary with the select word and the frequency
return word_dict
Essentially, this code starts with the first word in the string, then loops through the entire string to see how many times that word occurs and adds the frequency to the dictionary. Then it moves onto the second word and checks the entire string to see the frequency of that word and so on.
This isn't the most efficient way of solving this problem since you're looping through the word list as many times as there are words. So if there are 10 words in the words list, you're looping through the entire list 10 times.
I also don't recommend completely looking up solutions for challenges online. The greatest learning experiences come from struggling to solve the problem yourself and asking for guidance, rather than the solution. I hope this helps though and if you have more questions, I'd love to help :)!

walter fernandez
4,992 Pointshow can this code add a colon in the dictionary?

Robin Goyal
4,582 PointsWhen you print out the dictionary and you see the colons, those are automatically included.
There are two ways to create dictionaries in Python. The first way is when you want to create a dictionary and you know the dictionary key and values you want to add. This method uses the colon to define that a value is associated with a key.
person = {'name': 'Bob', 'age': 21}
The second way creates a key and value pair in an already existing dictionary but does not use a colon to do this.
person['gender'] = 'male'
Hopefully that makes sense!

walter fernandez
4,992 Pointsword_dict[sel_word] = count in the line above from the code what is the difference if I put word instead sel_word, between the brackets? I mean, both of them are index.