Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial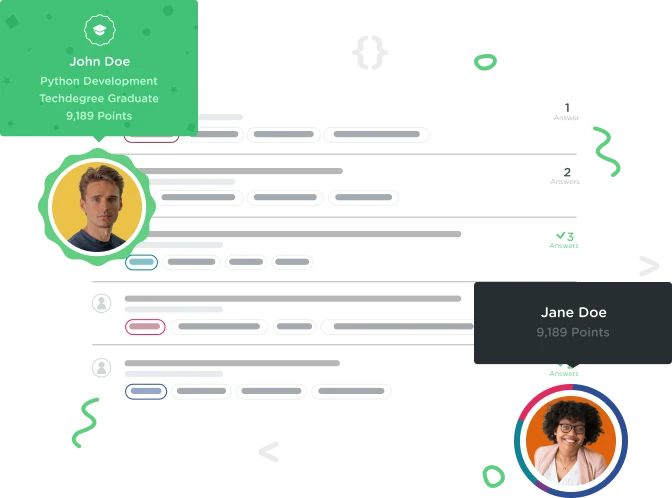

Logan Detering
1,953 PointsCan Someone tell me what Im doing wrong
Can't figure this Question out
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char theTile) {
int count = 0;
for(char tile: mHand.toCharArray()) {
if(tile == theTile) {
count++;
}
}
return count;
}
2 Answers
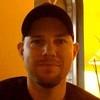
Jeremy Hill
29,567 PointsYou just need to add a closing curly brace to the end of your getTileCount method: '}'
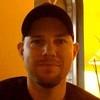
Jeremy Hill
29,567 PointsThe last one at the end of your code is for the class, you need one for the end of your method.
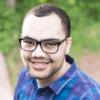
Philip Gales
15,193 PointsHere is what Jeremy was telling you to do. Whenever I have a lot of code, I will mark the closing curly braces so I know which ones are for the class and method.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char theTile) {
int count = 0;
for(char tile: mHand.toCharArray()) {
if(tile == theTile) {
count++;
}
}
return count;
}//end of getTileCount()
} //end of class
Simon Coates
28,694 PointsSimon Coates
28,694 PointsJeremy Hill is right. Keep in mind that the error messages it gives you (via preview on the challenge) are often pretty good clues about what and where the glitch is., and you can often google the phrase in question. "reached end of file while parsing" is usually going to mean a missing }.