Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial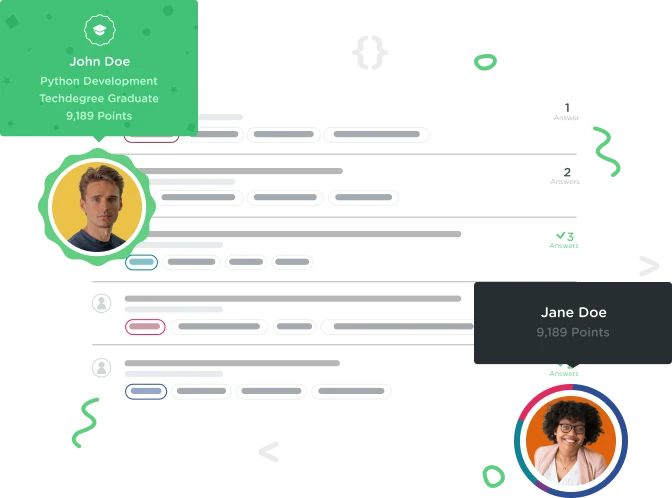

Ieva Salte
15,356 PointsCan someone tell me what is the other here? I cannot get this to work
Can some tell me what is this other object? Are we creating a new blogpost object called other?
package com.example;
import java.util.Date;
public class BlogPost implements Comparable{
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
public int compareTo(Object obj){
if (this.equals(obj)){
obj = ((BlogPost)obj);
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
}
1 Answer

Christian Andersson
8,712 PointsThe idea here is that you get an object as a parameter and you cast into a BlogPost object. Now you have 2 BlogPost objects; the current one (this
) and the one you got from the parameter. Since you now have 2 objects you can compare them and return accordingly.
So you'd want to the casting before and outside the if
clause because it's essential that you have 2 objects of similar type in order to compare them to one another.
Another error here is that you are trying to cast an Object
-type object into a BlogPost
object, but you store the result back into an Object
-type variable. So in essence you haven't done anything here. What you need to do is store the result into a BlogPost
variable (since that's what you are trying to cast it into).
Finally, what you want to do is compare the current objects (this
) mCreationDate
attribute with the parameterized objects' mCreationDate
attribute.
Here is what I tried:
public int compareTo(Object obj) {
BlogPost otherObj = (BlogPost) obj; //typecast the parameter into a BlogPost object
if (this.equals(otherObj)){
return 0;
}
return mCreationDate.compareTo(otherObj.getCreationDate()); //compare this.mCreationDate with the parameterized objects' mCreationDate value
}
Hope this helps! :)