Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial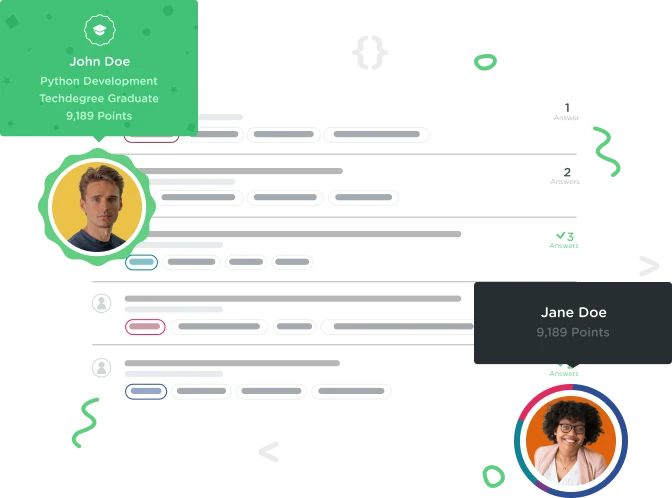
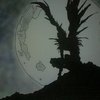
Lucas Santos
19,315 PointsCan someone tell me what's wrong with my code (Inheritance)
Im messing around trying to familiarize myself with inheritance and I keep getting an error in Workspace telling me "Cannot find symbol". Iv looked over my code many times and cannot figure out what's wrong.
Example.java
import java.util.*;
import java.io.*;
public class Example {
public static void main(String[] args) {
Cars superBike = new Motorcycle("Black", 100);
superBike.zoomZoom();
}
}
Cars.java
import java.util.*;
import java.io.*;
public class Cars{
public String mColor;
public int mSpeed;
public void takeOff(){
System.out.println("The car takes off the speed of " + this.mSpeed);
}
public Cars(String color, int speed){
mColor = color;
mSpeed = speed;
}
}
public class Motorcycle extends Cars{
public void zoomZoom(){
System.out.println("The " + this.mColor + " motorcycle takes off at the speed of " + this.mSpeed);
}
}
They are both in the same location as far as package goes. So I believe I do not need to import my Cars.java
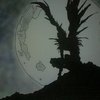
Lucas Santos
19,315 PointsHey Grigorij
It worked but it does not make sense. Everything that iv learned about casting in the Data Structure course says you can instantiate an instance like that because Cars is a Superclass of Motorcycle which is the subclass
So I had
Cars superBike = new Motorcycle("Black", 100);
Then you changed Cars to the subclass of Motorcycle like so:
Motorcycle superBike = new Motorcycle("Black", 100);
But what I had should have worked according to what I learned in the Java courses?
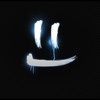
Grigorij Schleifer
10,365 PointsHey Lucas,
your Motorcycle class is inheriting ("extend") from the superclass Cars right. So if you instatializing a new Motorcycle object, this object is inheriting from the superclass too.
The Motorcycle object can use methods from the Motorcycle class or the super class Cars.
And look here:
http://stackoverflow.com/questions/2701182/call-a-method-of-subclass-in-java
This explains the topic very good
Grigorij
4 Answers

ginopino
16,320 PointsI think you have to declare a Motorcycle constructor that take those two arguments and call super(color, speed) in the first line. Cause, by default, you just have a basic constructor with no arguments.
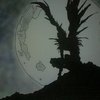
Lucas Santos
19,315 Pointstried that already and I end up getting a lot more errors,
public class Motorcycle extends Cars{
public void zoomZoom(){
System.out.println("The " + this.mColor + " motorcycle takes off at the speed of " + this.mSpeed);
}
public MotorCycle(mColor, mSpeed){
super(mColor,mSpeed);
}
}

ginopino
16,320 PointsWhat kind of errors? Can we see them?
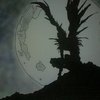
Lucas Santos
19,315 PointsTo see it better just open Workspae and create a new project and simply paste in my code.
Here are the errors after I try adding the super() on the Motorcycle constructor. Went from 2 errors to 8 after that.
./Motorcycle.java:5: error: invalid method declaration; return type required
public MotorCycle(mColor, mSpeed){
^
./Motorcycle.java:5: error: <identifier> expected
public MotorCycle(mColor, mSpeed){
^
./Motorcycle.java:5: error: <identifier> expected
public MotorCycle(mColor, mSpeed){
^
./Motorcycle.java:5: error: cannot find symbol
public MotorCycle(mColor, mSpeed){
^
symbol: class mColor
location: class Motorcycle
./Motorcycle.java:5: error: cannot find symbol
public MotorCycle(mColor, mSpeed){
^
symbol: class mSpeed
location: class Motorcycle
Example.java:8: error: cannot find symbol
superBike.zoomZoom();
^
symbol: method zoomZoom()
location: variable superBike of type Cars
./Motorcycle.java:6: error: cannot reference mColor before supertype constructor has been called
super(mColor,mSpeed);
^
./Motorcycle.java:6: error: cannot reference mSpeed before supertype constructor has been called
super(mColor,mSpeed);
^
8 errors

ginopino
16,320 PointsOh yes, you missed types
public MotorCycle(String mColor, int mSpeed){
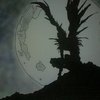
Lucas Santos
19,315 PointsThat fixed most of my errors but now there is only one error left and this is my code as of now.
import java.util.*;
import java.io.*;
public class Cars{
public String mColor;
public int mSpeed;
public void takeOff(){
System.out.println("The car takes off the speed of " + this.mSpeed);
}
public Cars(String color, int speed){
mColor = color;
mSpeed = speed;
}
}
public class Motorcycle extends Cars{
public void zoomZoom(){
System.out.println("The " + this.mColor + " motorcycle takes off at the speed of " + this.mSpeed);
}
public Motorcycle(String color, int speed){
super(color, speed);
}
}
Then in my Example.java
import java.util.*;
import java.io.*;
public class Example {
public static void main(String[] args) {
Cars superBike = new Motorcycle("Black", 100);
superBike.zoomZoom();
}
}
Now I am only left with one error and that is.
Example.java:8: error: cannot find symbol
superBike.zoomZoom();
^
symbol: method zoomZoom()
location: variable superBike of type Cars
1 error
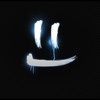
Grigorij Schleifer
10,365 PointsEspecially this explanation is good:
When dealing with inheritance/polymorphism in Java there are basically two types of casts that you see:
Upcasting:
Superclass x = new Subclass();
This is implicit and does not need a hard cast because Java knows that everything the Superclass can do, the Subclass can do as well.
Downcasting
Superclass x = new Subclass();
Subclass y = (Subclass) x;
In this case you need to do a hard cast because Java isn't quite sure if this will work or not. You have to comfort it by telling it that you know what you're doing. The reason for this is because the subclass could have some weird methods that the superclass doesn't have.
In general, if you want to instantiate a class to call something in its subclass, you should probably just instantiate the subclass to begin with -- or determine if the method should be in the superclass as well.
Thanks to artgon from stack overflow
Hope it helps a little bit ...
Grigorij
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHi Lucas,
what happens if you do it like this:
Tell us if it does the trick ..
Grigorij