Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial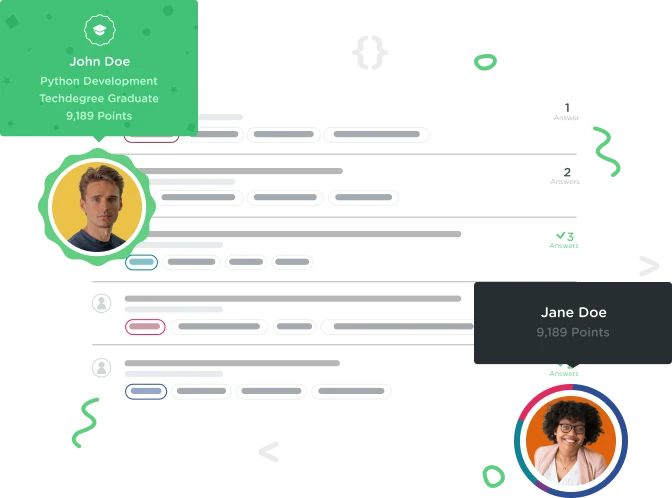

Karol Michalkowski
536 Pointscan someone tell what is wrong with my code in this challenge ? Thank you in advance.
Print "C# Rocks!" if language equals "C#" otherwise print language + " is not C#." So if I entered "Cheese" then "Cheese is not C#." would be printed to the screen.
Important: In each task of this code challenge, the code you write should be added to the code from the previous task.
CodeChallenge.cs
string language = Console.ReadLine(); ā if(language == "C#") { Console.WriteLine("C# Rocks!"); } else if(language == "Cheese"); { Console.WriteLine( "Cheese is not C#."); } else { Console.WriteLine("language + \"is not C#\""); }
ā
1 Answer
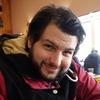
Eric M
11,546 PointsHi Karol,
Congrats on making it to this coding challenge: https://teamtreehouse.com/library/c-basics/perform/if-else-3
Just as an FYI - You can format your code by using three backticks followed by the language name, then your code, then three backticks to close it of.
```cs
Your code here
```
So, here's what we've got right now:
string language = Console.ReadLine();
if (language == "C#")
{
Console.WriteLine("C# Rocks!");
}
else if (language == "Cheese");
{
Console.WriteLine("Cheese is not C#.");
}
else
{
Console.WriteLine("language + \"is not C#\"");
}
Let's focus on the syntax errors first.
In your last Console.WriteLine
statement we want to print out whatever the user entered for language, followed by "is not C#".
Our variables don't get enclosed in brackets, only our literals (string literals are strings that are literally typed into the program, rather than read from a file or entered by the user).
We'll also add a fullstop at the end of our string to be exactly like the specification.
So we can change this line to be:
Console.WriteLine(language + " is not C#.");
On your else if
line you have a semi-colon after the condition. Conditions lead into blocks that are enclosed in curly braces, the statements within the block must be terminated with semi-colons, but we don't terminate the conditional statement, we just open the block, so we need to remove this semicolon.
Okay, now we've got:
string language = Console.ReadLine();
if (language == "C#")
{
Console.WriteLine("C# Rocks!");
}
else if (language == "Cheese")
{
Console.WriteLine("Cheese is not C#.");
}
else
{
Console.WriteLine(language + " is not C#.");
}
The thing is though - even though this will pass the challenge - we've got some redudant code in here. The else if
clause is not needed, because if someone types in "Cheese" our final else
clause will print the same thing as our else if
clause.
So, let's make this a bit neater by removing that branch:
string language = Console.ReadLine();
if (language == "C#")
{
Console.WriteLine("C# Rocks!");
}
else
{
Console.WriteLine(language + " is not C#.");
}
Now we've got something that passes the challenge and has a decent structure that makes sense.
Best of luck continuing through the C# track!
Cheers,
Eric