Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial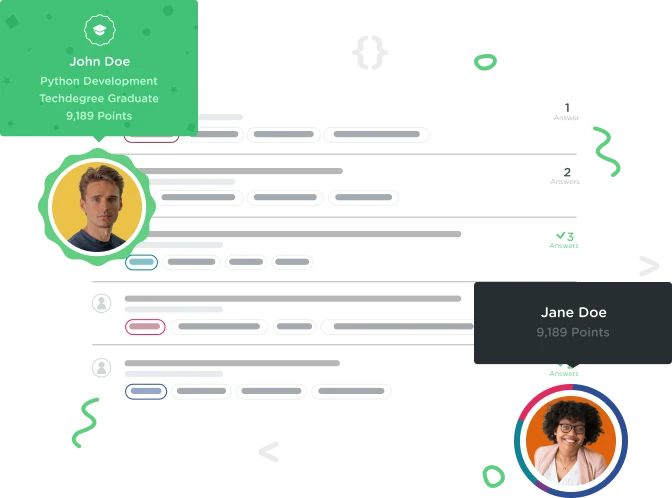

fmquaglia
11,060 PointsCan Swift Structs have optional stored properties?
Is there a way to define an optional stored property on a swift struct?
Something like:
struct Person {
var firstName : String
var lastName : String // not sure what the syntax would be
}
var john = Person(firstName: "John") // notice I'm passing only the firstName
6 Answers

Chris Shaw
26,676 PointsThe reason you got this error is because by default the constructor or init
method as it's called requires all stored properties to have an assigned value thus making them required even if they're optional, to get around this you simply need to create your own init
method which accepts only the parameters you need it to.
See the following.
struct Person {
var firstName: String
var lastName: String?
init(firstName: String) {
self.firstName = firstName
}
}
var john = Person(firstName: "John")
if let lastName = john.lastName {
println("\(john.firstName)' last name is \(john.lastName)")
} else {
println("\(john.firstName) doesn't have a last name")
}
Happy coding!

Hikarus Guijarro-Fayard
86 Pointsfor me, just including the default values in the struct's init worked. Here's an example I'm working on:
struct DummyMatch {
var gender: Bool
var name: String
var location: String
var status: MatchStatus
var scheduled: String
init(gender: Bool = false, name: String, location: String = "San Francisco", status: MatchStatus = .listed, scheduled: String = "15/05/1@17:30") {
self.gender = gender
self.name = name
self.location = location
self.status = status
self.scheduled = scheduled
}
}
var match = DummyMatch(name: "Mary")
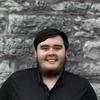
Michael Hulet
47,913 PointsYes, they can, but you must set an initial value on them, or make an explicit designated initializer. I think what you'd want is something like this:
struct Person {
var firstName: String
//Notice the ? below
var lastName: String? = nil
}

Chris Shaw
26,676 PointsYou don't actually need to assign nil
as an explicit value as ?
implies a nil
value by default thus the following will suffice.
var lastName: String?

fmquaglia
11,060 PointsIt seems that even declaring the stored parameter as optional, and/or setting a default value, you need to declare all the stored parameters when initialising an instance.
See this playground screenshot:
And the same for the version suggested by Chris Upjohn
and the fix for the error:
Thanks anyway guys!

fmquaglia
11,060 PointsChris Upjohn, please post your last answer as top-level comment on the thread, so I can choose it as "Best Answer". You seriously rock, my friend!

fmquaglia
11,060 PointsWell, at the end the way to declare certain stored values when initialising an struct, and make the other optional is to declare custom initialisers and overload them
struct Person {
var firstName : String
var lastName : String? = nil
init(firstName: String) {
self.firstName = firstName
}
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
}
var john = Person(firstName: "John", lastName: "Smith")
var jane = Person(firstName: "Jane")