Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial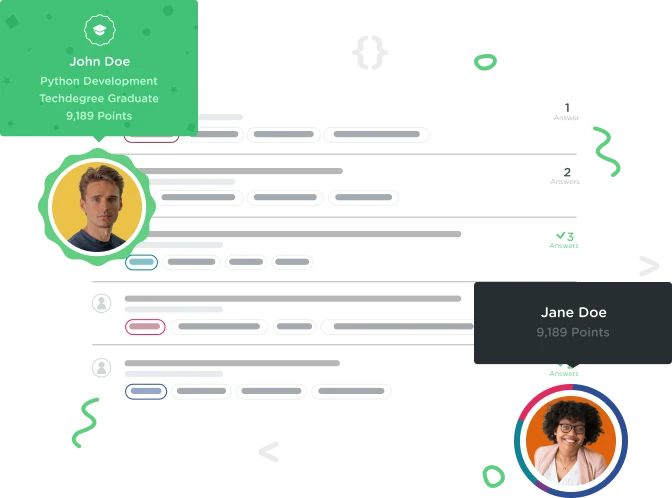

Patrick Strauss
587 PointsCan´t figure out last exercise! Please help!
For task 1 I modified the drive method to show:
public void drive(int laps) { lapsDriven += laps; barCount -= laps; if (barCount < 0) { throw new IllegalArgumentException("Not enough battery!!"); } }
I get an error method saying that I must throw the exception before changing the barCount and lapsDriven fields.
Mathwise and logically it seems like the code would work but I must be confused. Could someone please explain to me why this wouldn´t work and how I should change it for it to do so??
Thanks!!
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
lapsDriven += laps;
barCount -= laps;
if (barCount < 0) {
throw new IllegalArgumentException("Not enough battery!!");
}
}
}
3 Answers

Ionut Mitrita
5,546 PointsHey Patrick,
If an exception is being thrown in a method, you also have to specify this in the definition of the method. Also, regarding this: "I get an error method saying that I must throw the exception before changing the barCount and lapsDriven fields." The issue with you code is that you are changing the global variables and THEN checking if it's a problem and throwing an exception, but the global variables are already changed. You need to check if it's ok first, then change the global variables. Therefore the correct code would be:
public void drive(int laps) throws Exception{ if (barCount < laps) { throw new IllegalArgumentException("Not enough battery!!"); } lapsDriven += laps; barCount -= laps; }

JD Lemon
3,771 PointsHey Patrick,
Not sure if I'm totally on point here but my understanding when you enter into the method is to first check if the battery level is greater than 0 (so you can drive a lap since it takes 1 battery level to drive 1 lap). So when you enter the method, you check the battery level, then drive a lap, then decrement the battery. If the battery was already at 0 when you entered the drive method, then it would increase the laps, and decrease the battery to -1 before throwing the exception.

Patrick Strauss
587 PointsHey guys, thank you so much for your kindness in clearing up my confusion. I solved it now (with your assistance). I´m sorry it took me a while to respond to this, but I really appreciate all your help! Issue resolved! :)
Tomasz Baranczuk
7,055 PointsTomasz Baranczuk
7,055 PointsI think the problem is here
if (barCount < 0)
From this I understand that you can not drive any more when barCount is equel to -1. When it is 0 you can steel drive. For me it should be: barCount <1 or barCount <= 0