Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial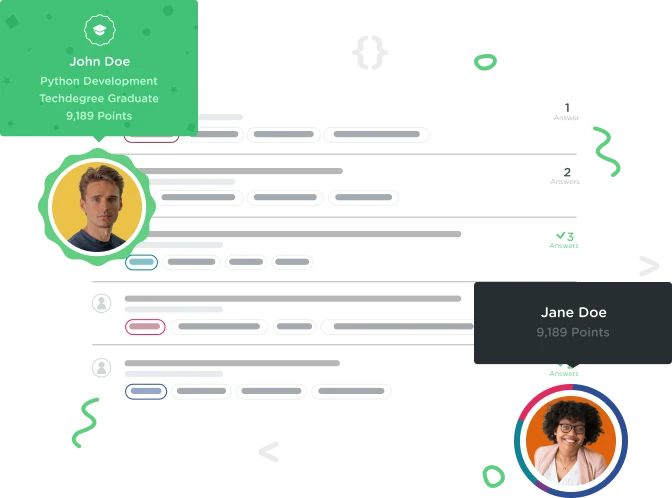
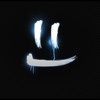
Grigorij Schleifer
10,365 PointsCan´t pass the last challange in Harnessing the Power of Objects course
Please help !!! I am stuck on this challenge and can´t figure out the problem ....
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
try {
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
kart.drive();
throw new IllegalArgumentException();
} catch (IllegalArgumentException);
System.out.println ("not enough juice to drive");
}
}
5 Answers

Dan Johnson
40,533 PointsThe drive method will be throwing the exception, so you don't need to throw it yourself.
Your catch should have a block associated with it, and don't forget to give the exception variable a name so you can reference it. Here's the basic Try-Catch structure:
try {
//...
}
catch(IllegalArgumentException e) {
//...
}
Then you just need to call the getMessage method on the exception that was thrown and print that out.
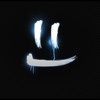
Grigorij Schleifer
10,365 Pointspublic class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
try {
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
} catch (IllegalArgumentException a) {
kart.drive();
a.getMessage();
System.out.printl (a);
}
}
}

Dan Johnson
40,533 PointsMove kart.drive()
back into the try block since that's the code you're checking, and then pass the result of getMessage to println instead of the exception itself.
Should be good to go after that.

Dan Johnson
40,533 PointsAdded code tags to your post.
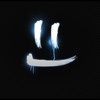
Grigorij Schleifer
10,365 PointsAnother question ... how to add code tags to code ????

Dan Johnson
40,533 PointsAbove the "Post comment" or "Post answer" button you'll see a link named Markdown Cheatsheet. That will list all the formatting options.
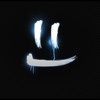
Grigorij Schleifer
10,365 PointsThe correct result looks like this ... thanks a lot :)))))
public class Main {
public static void main(String[] args) {
GoKart kart = new GoKart("yellow");
try {
kart.drive();
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
} catch (IllegalArgumentException a) {
String message = a.getMessage();
System.out.println (message);
}
}
}
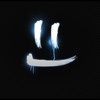
Grigorij Schleifer
10,365 PointsGreat !
Thanks Dan !!! Greetings from rainy germany :)