Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial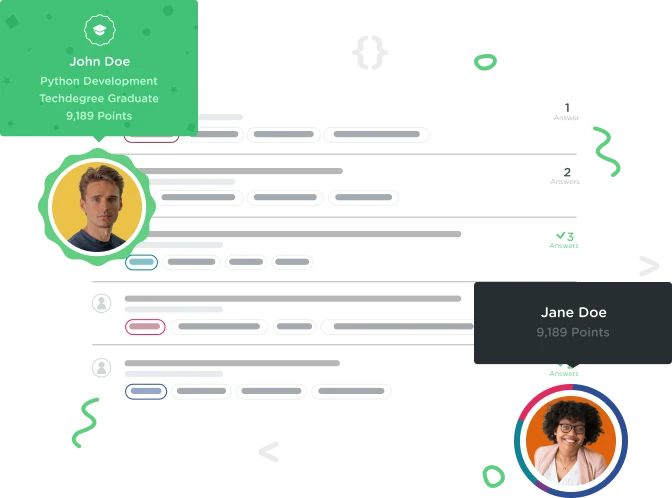
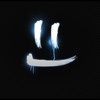
Grigorij Schleifer
10,365 PointsCan´t solve the Sets challenge
Here is my code in the Blog class:
public Set<String> getAllAuthors(){
Set<String> allPosts = new TreeSet<String>();
for(String word: getPosts()){
if(word.equals(mAuthor))
allPosts.getAuthor(word);
}
}
return allPosts;
}
Here ist the error:
./com/example/Blog.java:19: error: class, interface, or enum expected
public Set getAllAuthors(){
^
./com/example/Blog.java:21: error: class, interface, or enum expected
for(String word: getPosts()){
^
./com/example/Blog.java:24: error: class, interface, or enum expected
}
^
./com/example/Blog.java:27: error: class, interface, or enum expected
}
^
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
4 errors
What i am doing wrong????
5 Answers

Mat Sanders
4,819 Pointsshot in the dark, just noticed it right off the bat...
for(String word: getPosts()){
if(word.equals(mAuthor)){ <--bracket
allPosts.getAuthor(word);
} <--bracket
}
Like Miroslav Kovac said would need more code to see more.
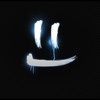
Grigorij Schleifer
10,365 PointsThis worked for me:
public Set<String> getAllAuthors(){
Set<String> allPosts = new TreeSet<String>();
for(BlogPost word: getPosts()){
String postAuthors = word.getAuthor();
allPosts.add(postAuthors);
}
}
return allPosts;
}

Dennis Van Laarhoven
988 Pointsit does not for me :-(

Chris Atchley
18,468 PointsThere looks to be an extra closing brace before your return statement.
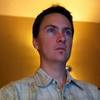
ryanjones6
13,797 Points<p>Grigorij Schleifer example is on the mark except for the extra ending curly bracket before the return statment.</p>
public Set<String> getAllAuthors(){
// <Create a new temporary ordered Set that contains the type String>
Set<String> allPosts = new TreeSet<String>();
// <iterate through each BlogPost>
for(BlogPost word: getPosts()){
// <adds author from each BlogPost to the temporary ordered Set: allPosts>
allPosts.add(word.getAuthor());
}
// <returns all post authors as an ordered set>
return allPosts;
}
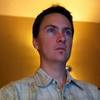
ryanjones6
13,797 PointsThanks for the responses from everyone. I kind of forgot about this post.
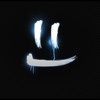
Grigorij Schleifer
10,365 PointsNow I am here:
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors(){
Set<String> allPosts = new TreeSet<String>();
for(BlogPost word: getPosts()){
if(word.equals(author)){
allPosts.getAuthor(word);
}
}
return allPosts;
}
}
and get this error:
./com/example/Blog.java:20: error: cannot find symbol
if(word.equals(author)){
^
symbol: variable author
location: class Blog
./com/example/Blog.java:21: error: cannot find symbol
allPosts.getAuthor(word);
^
symbol: method getAuthor(BlogPost)
location: variable allPosts of type Set
Note: JavaTester.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
2 errors
Why the compiler can´t find the author and the getAuthor method? Or am I on the wrong path ???
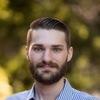
Ronald Williams
Java Web Development Techdegree Graduate 25,021 Pointsimport com.example.BlogPost;

Mat Sanders
4,819 PointsNot sure the other issue, but haven't done this course yet, but a treeset has no "getAuthor". But you might have set that somewhere else. Like I said haven't done this course so really another shot in the dark.
Miroslav Kovac
11,454 PointsMiroslav Kovac
11,454 PointsHello, can you please share the whole code. I think that you are missing some bracers '{' or '}'. Or maybe your method is not in the class.