Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial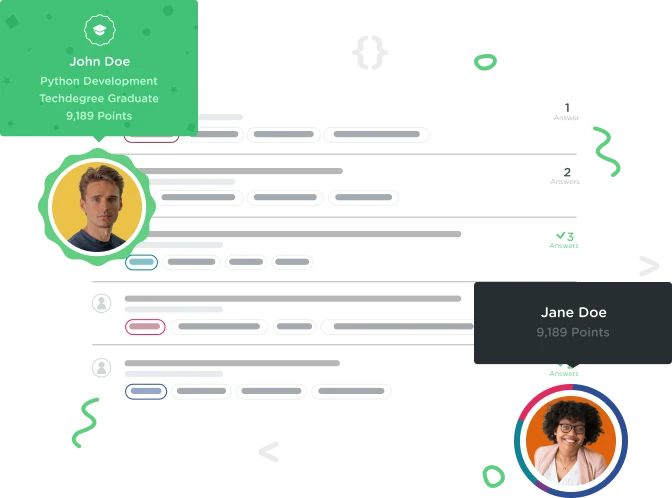
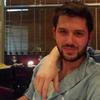
Nir Frank
3,578 PointsCan this code be improved?
I'm trying to build my own little program in order to practice some concepts learned so far in this course, and in the "Intro to Programming" course.
My program will ask for a user's first and last name, their current city of residence, and the weather - and will then return them in addition to some manipulation of the data (length of name, city in all caps, weather in all lowercase). If the user doesn't enter anything in any given prompt, they will be alerted and asked to input that information again.
My program works, but I was wondering in what ways it can be improved (considering what I've learned so far in the two courses). Can it be consolidated? Can I use a better method to force the user to input a valid string? Etc.
Here is my code:
/*
Take a user's name, current city of residence, weather, and print back their name's length + their city in uppercase + their weather in lowercase.
If a user doesn't enter a string to either one of these, pop up an alert telling them so.
*/
var firstName = prompt("What is your first name?");
while (firstName.length === 0) {
alert("Please enter your first name");
firstName = prompt("What is your first name?");
};
var lastName = prompt("What is your last name?");
while (lastName.length === 0) {
alert("Please enter your last name");
lastName = prompt("What is your last name?");
};
var fullName = firstName + " " + lastName;
var currentCity = prompt("What city do you currently reside in?");
while (currentCity.length === 0) {
alert("Please enter the city you currently reside in!");
currentCity = prompt("What is the city you currently reside in?");
};
var weather = prompt("What's the weather like?");
while (weather.length === 0) {
alert("Please enter the weather!");
weather = prompt("What's the weather like?");
};
document.write("<h1>Your name is <b>" + fullName + "</b>! It is <i>" + fullName.length + "</i> characters long! </h1>");
document.write("<h2>You currently live in " + currentCity.toUpperCase() + ". </h2>");
document.write("<h3>It looks like the weather over there is " + weather.toLowerCase() + "!");
Thanks!
2 Answers

Andrei Fecioru
15,059 PointsThe first thing that strikes me about your code snippet is the amount of code repetition. You should strive to follow the DRY principle (Don't Repeat Yourself) and factor out the data acquisition phase into a separate function. Here's my take on it:
/*
Take a user's name, current city of residence, weather, and print back their name's length + their city in uppercase + their weather in lowercase.
If a user doesn't enter a string to either one of these, pop up an alert telling them so.
*/
function answerQuestion(question, retryMessage) {
var answer = prompt(question);
while (answer.length === 0) {
alert(retryMessage);
answer = prompt(question);
};
return answer;
}
var firstName = answerQuestion("What is your first name?", "Please enter your first name");
var lastName = answerQuestion("What is your last name?", "Please enter your last name");
var fullName = firstName + " " + lastName;
var currentCity = answerQuestion("What city do you currently reside in?",
"Please enter the city you currently reside in!");
var weather = answerQuestion("What's the weather like?",
"Please enter the weather!");
document.write("<h1>Your name is <b>" + fullName + "</b>! It is <i>" + fullName.length + "</i> characters long! </h1>");
document.write("<h2>You currently live in " + currentCity.toUpperCase() + ". </h2>");
document.write("<h3>It looks like the weather over there is " + weather.toLowerCase() + "!");
William Li
Courses Plus Student 26,868 PointsCan I use a better method to force the user to input a valid string?
Yes, you can, by using Regular Expression to validate the input strings; But Regular Expression
is considered somewhat an advanced topic in JavaScript and you probably haven't learned it yet from lectures so far.