Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial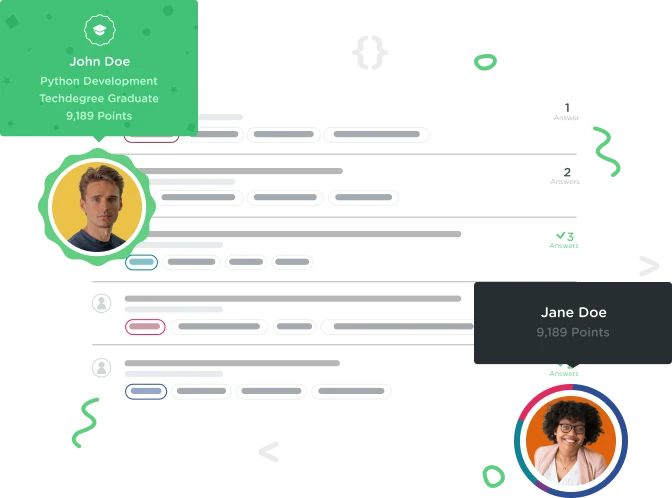

Andrey Serebryanskiy
5,756 PointsCan this piece of code be replaced with something functional?
Hey!
I have a simple Minesweeper game. When I create a field for it, I go through each cell of two-dimensional tile array and put a new Tile there. X and y are important because they are further used to add a tile to a GridPane.
xTiles - is number of tiles horizontally
yTiles - vertically.
The last argument in new tile creation determines if this tile would have a bomb.
Tile[][] tiles = new Tile[xTiles][yTiles];
for(int y = 0; y<yTiles; y++) {
for(int x = 0; x<xTiles; x++) {
tiles[x][y] = new Tile(x, y,Math.random() < 0.15);
}
}
Can this massive piece of code be replaced with more succinct and understandable stream?
2 Answers

Thomas Nilsen
14,957 PointsYou can probably do something like this:
IntStream.range(0, xTiles)
.forEach(r -> IntStream.range(0, yTiles)
.forEach(c -> array[r][c] = new Tile(r, c)));
or something like this:
Tile[][] array = IntStream.range(0, xTiles)
.mapToObj(r -> IntStream.range(0, yTiles)
.mapToObj(c -> new Tile(r, c))
.toArray(Tile[]::new))
.toArray(Tile[][]::new);
Andrey Serebryanskiy
5,756 PointsAndrey Serebryanskiy
5,756 PointsThank you, Thomas, you've provided a very beautiful decision.