Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial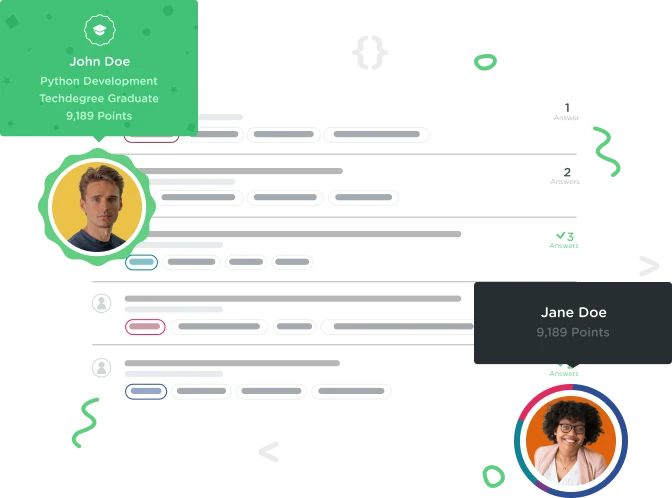
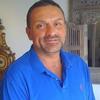
Marco Aniello
3,297 PointsCan we learn integrating Swift with Facebook and/or Parse?
Hello Team Treehouse!
I would like to suggest a Swift course that would cover how to integrate Swift with Facebook and/or Parse.
So many modern apps, games and websites allow people to just login with Facebook with the touch of a button and this would be wonderful!
With Parse you have an older course on how to do it with Objective C, any chance we could do an updated one with Swift?
Thanks!
5 Answers
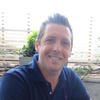
Gareth Gomersall
2,890 PointsThis took me around a week to finally figure out how to integrate Facebook log in/sign up with Parse as a backend.
I followed the instructions on getting Parse setup and testing the connection from an app to the backend on Parse, i.e. from the docs located here - https://www.parse.com/apps/quickstart#parse_data/mobile/ios/native/existing
I also followed the Facebook docs on setting up your app on Facebook and importing/adding the necessary info into your Xcode project, i.e. from the docs located here - https://developers.facebook.com/docs/ios/getting-started , up to the point where is says "Start Coding"
Now the Facebook info in the docs above are all in obj-c, and the parse documentation for login (currently) is not for swift 1.2 - so to me it's redundant. I did however, after a few days figure out how to achieve my goal of logging my user into my app via Facebook.
Now here comes the fun stuff! I'll let you know my version of Xcode and swift so that you can make sure you have the same as me to make this work.
- Xcode v. 6.3.1
- Swift v. 1.2
- iOS SDK 8.3
Now you want to use a bridging header file for this to work, so in your bridging header file make sure you have declared your necessary SDKs, mine as are follows (the important ones are the first 4, the FBSDKShareKit, if it you want to allow sharing from within your app to Facebook, but that's a whole different set of instructions and DOES NOT relate to this answer):
#import <Parse/Parse.h>
#import <Bolts/Bolts.h>
#import <ParseFacebookUtilsV4/PFFacebookUtils.h>
#import <FBSDKCoreKit/FBSDKCoreKit.h>
#import <FBSDKShareKit/FBSDKShareKit.h>
next you need to initialise the Parse Facebook utils (remember i'm using ParseFacebookUtilsV4), so head over to your AppDelegate.swift file and do the following:
- Find the function below (normally at the top of the file) -
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool
and add the following line -
PFFacebookUtils.initializeFacebookWithApplicationLaunchOptions(launchOptions)
- Still in the AppDelegate.swift file you now have to declare a new function.
The function is as such -
func application(application: UIApplication,
openURL url: NSURL,
sourceApplication: String?,
annotation: AnyObject?) -> Bool {
return FBSDKApplicationDelegate.sharedInstance().application(application,
openURL: url,
sourceApplication: sourceApplication,
annotation: annotation)
}
That's all you need to do to your AppDelegate.swift file, now head over to your View controller and do the following steps.
Now my ViewController is called StartViewController, but it could be called anything depending on how you setup your project. The tutorials on Facebook for login have two methods, one is to have a button automatically generated by Facebook, and the other way is to create your own button with an IBAction associated to it. I've gone for the latter, and have created my own button.
- So all i needed to do was to connect the button from the corresponding XIB file to my ViewController. which should look like this once you've connected it -
@IBAction func didTapFacebookConnect(sender: AnyObject) {
}
Your function of the button may be called something else, but i've named mine, didTapFacebookConnect.
- Next you need to declare the permissions that you app will use from a Facebook profile. I chose to use "public_profile", "email", and "user_friends". You can use others, some need approval by Facebook and some don't. The ones i have used don't need any approval from Facebook.
So to declare the permission i simply added the following line under my connected button -
var permissions = [ "public_profile", "email", "user_friends" ]
- Now comes the bit where you need to connect everything up to Parse and allow your user to login in and/ or sign up to the app.
Add the following lines just under the permission that you've setup -
PFFacebookUtils.logInInBackgroundWithReadPermissions(permissions, block: { (user: PFUser?, error: NSError?) -> Void in
if let user = user {
if user.isNew {
println("User signed up and logged in through Facebook!")
} else {
println("User logged in through Facebook!")
}
} else {
println("Uh oh. The user cancelled the Facebook login.")
}
})
So all in, that block of code in your ViewController should look something like this -
@IBAction func didTapFacebookConnect(sender: AnyObject) {
var permissions = [ "public_profile", "email", "user_friends" ]
PFFacebookUtils.logInInBackgroundWithReadPermissions(permissions, block: { (user: PFUser?, error: NSError?) -> Void in
if let user = user {
if user.isNew {
println("User signed up and logged in through Facebook!")
} else {
println("User logged in through Facebook!")
}
} else {
println("Uh oh. The user cancelled the Facebook login.")
}
})
}
I hope that this helps anyone stuck on this kind of thing, as trying to find a solution was very frustrating for me. If you need anything else related to this, or if you get stuck, feel free to hit reply to this answer.
Gareth
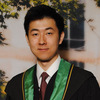
Jason Gong
4,537 PointsI've been trying to do the same! A course would be really awesome. Since there isn't one yet, I've been trying to piece together how to make it work. I found these resources useful:
General guidance on how to get the SDK set up and test it out. Parse actually has a built in function that makes the sign up really easy. Getting the button to look nice and work like FBLoginView takes a bit of work though. https://parse.com/docs/ios_guide#fbusers/iOS
This is a ObjC version of the tutorial. Might take a bit of googling to get the functions in swift, but it's easy to follow. https://www.youtube.com/watch?v=tFF0dEod5XQ
Here is a tutorial on making a login screen with a navigation controller. Good to set up screens that link together for users wanting to sign up vs. to log in. http://dipinkrishna.com/blog/2014/07/login-signup-screen-tutorial-xcode-6-swift-ios-8-json/
Hope this helps! I'm still trying to learn as well. Making a login screen seems so much harder than I thought it'd be.
Jason
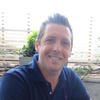
Gareth Gomersall
2,890 Points@Jasongong Did you get this working yet?
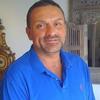
Marco Aniello
3,297 PointsJason thank you so much, those links are a big help to me. I really appreciate those suggestions so I can get moving forward on my app. I dont know why I didnt see those before in my searches. thanks!
Marco

Wilson Muñoz
16,913 PointsI second this, i think it would be great the learn it. Parse maybe as a Workshop? Just an idea.
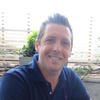
Gareth Gomersall
2,890 PointsWilson Muñoz did you find a way to do this?

Wilson Muñoz
16,913 Pointshttps://www.youtube.com/watch?v=7sL47vpWTMU i kinda used this.
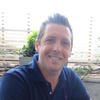
Gareth Gomersall
2,890 PointsOk it doesn't seem to include using Parse in that tutorial... I've managed to work out how to do this with Parse, so i'll post an answer down below.
Gareth Gomersall
2,890 PointsGareth Gomersall
2,890 Points@marcoaniello Did you get this working yet?