Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial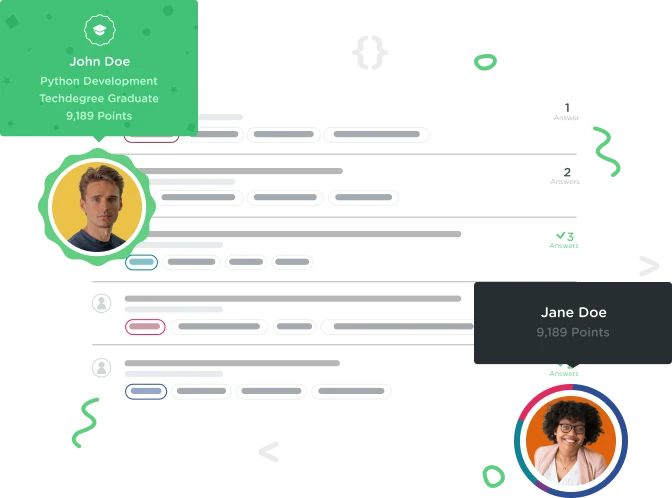
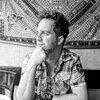
jlampstack
23,932 PointsCan we nest the prototype?
To me, even though the objects are now sharing the same prototype object, it's still floating.
Is it possible to nest the Dice.prototype.roll function?
3 Answers
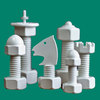
Steven Parker
231,248 PointsThere are two issues that come to mind. First, you're not just adding the "roll" method to the "Dice" class, but to every object in the system. And by making the prototype assignment inside the constructor, the assignment will be re-done every time a new "Dice" object is created. Since it overwrites the previous definition it won't use more memory, but it takes unnecessary execution time.
I wouldn't consider code that establishes prototype functions to be "floating around" if it is always kept adjacent to the class constructor definition.
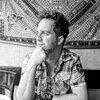
jlampstack
23,932 PointsThis is the answer I was looking for. Thanks!
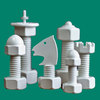
Steven Parker
231,248 PointsIf you're asking if you can define the "roll" function inside the "Dice" constructor, then yes you can. But one disadvantage of doing that is that every instance of the class will have it's own separate copy of the "roll" function, but if you define it as part of the prototype, all instances will share the same function.
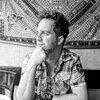
jlampstack
23,932 PointsSteven Parker , thanks for your reply. I understand the part your were explaining. I provided an example of what I'm trying to achieve in a thread below. Can you let me know if the example used below is acceptable? Thanks!
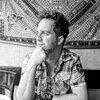
jlampstack
23,932 PointsThrough a little research, trial and error, we can nest the prototype, using Object.prototype.roll
instead of Dice.prototype.roll
function Dice(sides) {
this.sides = sides;
Object.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
}
var dice = new Dice(6);
var dice10 = new Dice(10);
Now the following code on the line below returns true
and i would assume it doesn't waste memory.
console.log(dice.roll === dice10.roll);
Since this is nested inside of the Dice function, it's nolonger floating around, and for me personally, I find it easier to find since it's grouped together.
Are there any downsides in nesting the prototype this way? Or is this acceptable?

Clayton Perszyk
Treehouse Moderator 48,850 PointsYou might look into ES6 Class construct, if you want to have the methods nested.

Emmanuel Jimenez
21,666 PointsI don't recommend you to try this and this is why:
- Every object or nearly every object that you create in Javascript are instances of Object.
That being said, if you do this:
Object.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
You are assigning the roll() function to every object that you create in Javascript. If you create another completely different object, this new object will have this method too.
Here is an example:
//Dice Object
function Dice(sides) {
this.sides = sides;
Object.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
}
//New different object
function NewObject() {
//
}
var dice = new Dice(6);
var newObject = new NewObject();
console.log(dice.roll === newObject.roll);
You will see that the console will return TRUE. So I don't recommend you to try this unless you want all your objects to share the same method.
Clayton Perszyk
Treehouse Moderator 48,850 PointsClayton Perszyk
Treehouse Moderator 48,850 PointsCould you elaborate more on what you mean? Thanks