Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial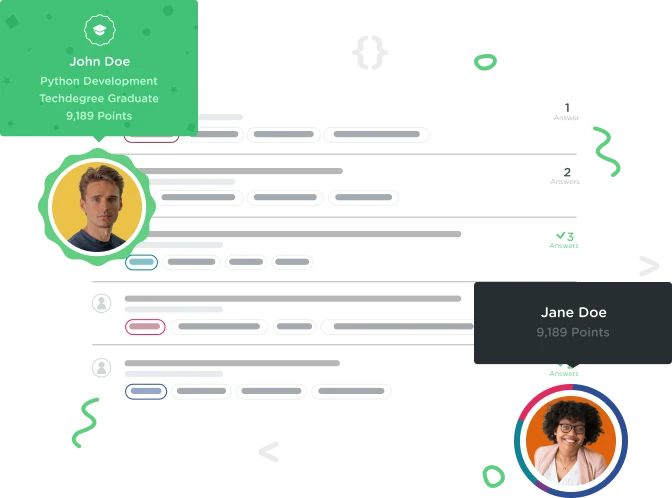

Placid Rodrigues
12,630 PointsCan we save the drawings?
Can we save the drawings created on the canvas? Can someone tell how should we go about it?
2 Answers
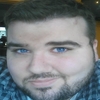
Marcus Parsons
15,719 PointsHey Placid Rodrigues,
There are a couple ways to save the image from a canvas. The easiest way is to just right click the canvas and select "Save Image As..." (or something similar). You can check out my paint app here. If you wanted to add a button for users on mobile device, tablet, etc., it is easy to do that as well.
Let's say you have a button:
<button id="saveDrawing">Save Drawing</button>
And when the user clicks this button, it will pop up with a window that has the filename pre-filled out for them (they can of course change it):
//From my own paint program:
$("#saveDrawing").click(function () {
//Select the canvas with id of "canvas" and convert it into a data URL
//The MIME type supported by almost every browser in existence is PNG
//However, other MIME types may be available depending on the browser
var dataURL = document.getElementById("canvas").toDataURL("image/png");
//Create new anchor element
var imga = document.createElement("a");
//Make its href value be the data URL received from the canvas
imga.href = dataURL;
//This sets the default filename
imga.download = "myImage.png";
//Append the anchor to the body
document.body.appendChild(imga);
//Activate its click function, which will activate the data URL and allow the user to save the image
imga.click();
});

Love Brynge
12,635 PointsActually I solved it!! I had forgotten to add an ID to the canvas :)
Placid Rodrigues
12,630 PointsPlacid Rodrigues
12,630 PointsThanks Marcus Parsons and congrats on great job on your project !!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnytime, Placid! And I appreciate that very much. Good luck on your projects! :)
Love Brynge
12,635 PointsLove Brynge
12,635 PointsHi! and thanks for the help!
I cant get this to work though. I get this error message: Uncaught TypeError: Cannot read property 'toDataURL' of null
here is my code:
Do you have any ideas on what might be wrong?