Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial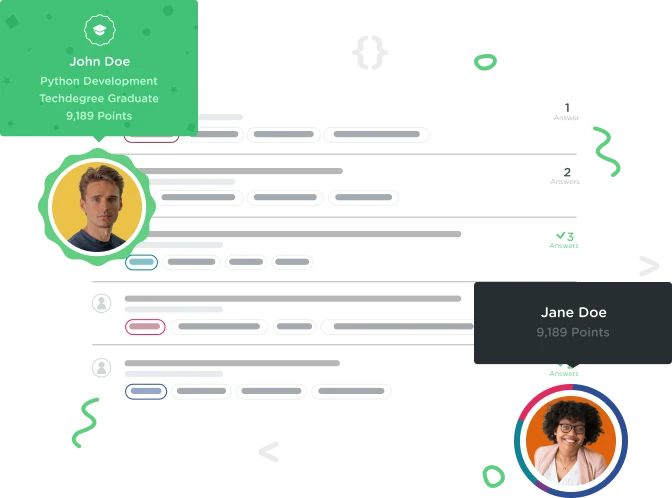
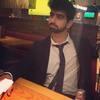
Faisal Rana
Python Web Development Techdegree Student 2,008 PointsCan we use a same variable multiple times ?
"Craig" used a variable with same name multiple times i.e number_of_characters = len(praise) number_of_characters = len(advice) number_of_characters = len(advice2)
Using same variable multiple times with different values & it's working. How ?? I thought it's impossible. Because one variable name is associated to one specific value. But here it isn't. I didn't quite get that part ?
2 Answers
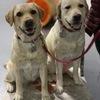
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Faisal,
It's totally fine to change the value of a variable (at least, it is in most languages, like Python). Indeed, we see it all the time, like in the following code:
x = 1
x = x + 1
We start by assigning the value 1 to x
. On the next line, we read the current value for x
, add 1 to it, and then assign that result back to x
. Indeed, this kind of action is so common that almost every language has a shortcut operator for it:
x = 1
x += 1
One way to understand why this is valid is to imagine that a variable is a temporary link to an object (in the examples above, a number, but in principle any kind of object in Python). You can always point that link to a new value. For example:
word = "hello"
word = "world"
In the first line, we told Python to link a string object of the form "hello" with the variable name 'word'. In the next line we told Python to change that link so that instead of referring to the "hello" object, it now refers to the "world" object.
Similarly, some types of object are "mutable", which means they can change. An example of this in Python is a list. So you might have something like this:
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # will print [1, 2, 3, 4]
You might not have got to lists and append
yet, but basically this is a method that appends a new value to the end of an existing list. So we create a list [1, 2, 3]
and refer to it with the variable named my_list
.
We then modify the list by adding 4 to the end of it.
my_list
still refers to the same list, but the list itself has now changed.
As far as Python is concerned, my_list
still points at the same object it did before. This is subtly, but importantly, different to the string example where my_list
ended up pointing at a different object than it did before.
Now that we've got the mechanics of variables out of the way, you make a good observation: often we do want to hold on to a value. This means that it is usually good practice to store the results of a destructive operation in a different variable than the one with the prior value, especially if we think we will need it later.
The only caveat to this is that as long as a variable continues to be in scope (a whole other concept that will become clear soon enough), the value referenced by the variable must stay in memory. When we're dealing with small things like individual numbers, or short strings, this is no big deal, but it's easy to imagine working with much larger objects, e.g., a video file, or a huge database, where we risk running out of memory and we want to free up the memory we were using as quickly as possible which we can do by assigning the edited or refined value back to the same variable:
Hope that makes sense,
Cheers
Alex

Baruch Zeif
7,654 PointsIt is possible to reassign the value of a variable. It works fine, but whatever previous value you had is gone. I think that when writing an actual program you wouldn't necessarily want to do that as you might need to use that value again for something else. I think for the purpose of the video he did it that way so that each block is basically the same and it is easier to see it's transition into being a function.
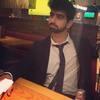
Faisal Rana
Python Web Development Techdegree Student 2,008 PointsThanks mate ! Now I understood.
Faisal Rana
Python Web Development Techdegree Student 2,008 PointsFaisal Rana
Python Web Development Techdegree Student 2,008 PointsThanks Alex. It makes sense now. Appreciated.