Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial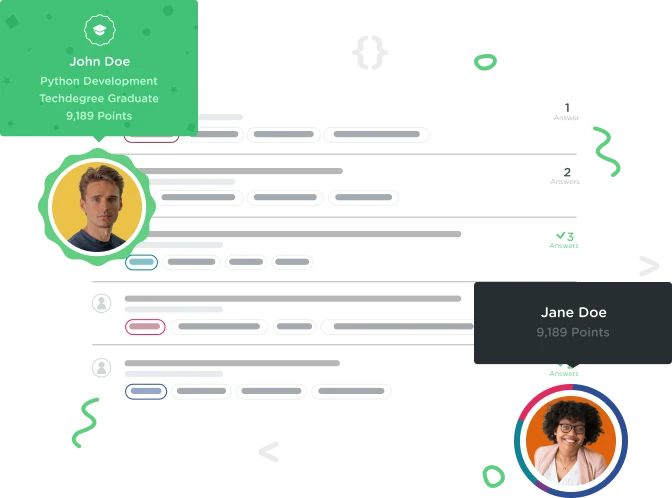

VICTORIA DENNIS
Full Stack JavaScript Techdegree Student 3,679 PointsCan you add an index of one array into another without the array taking each letter separately?
In the code below, I tried to push and, on this occasion, spread the first index of the array into a new array. The idea was - whatever question was being asked currently would be put into this array.
``` else if (answer === questions[i][1]){
console.log (`correct`);
counter++;
correct = [...questions[i][0]];
console.log(correct);```
However, when I try this, the 'correct' array is filled with the question, but each letter of the question is a different index. It takes the index of 'questions' and breaks it up into individual letters and indices and puts them into 'correct'. This is some of what comes out:
35) ["S", "t", "i", "c", "k", "i", "n", "g", " ", "y", "o", "u", "r", " ", "n", "o", "s", "e", ",", " ", "m", "a", "k", "e", "s", " ", "y", "o", "u", " ", "w", "h", "a", "t", "?"]
Then when trying to make the ordered lists using (below), it only displays one letter for each ordered list, even if multiple questions were wrong or right.
``function createList (arr) {
let item ='';
for (let i = 0; i < arr.length; i++){
item +=
<l1>${arr[i]}</l1>`;
return item;
}
}
document.querySelector('main').innerHTML = <h1>you got ${counter} correct</h1>
You got these questions right!
<ol>
${createList(correct)}
</ol>
You got these questions wrong!
<ol>
${createList(wrong)}
</ol>
;```
I feel like the places of error in my code is the consolidation into the second array, and the displaying it. The code worked for the first part of the assignment, but when it came to the lists, it fell apart. Is this because my code can't be adapted like this, or do I not know something?
This is my full code.
const questions = [
['How many lines are in the sea?', '1'],
['At max effort, can you breathe?', 'no'],
['Sticking your nose, makes you what?', 'sticky']
];
let message;
let counter = 0;
let correct = [];
let wrong = [];
for (let i = 0; i<questions.length; i++){
let answer = prompt(questions[i][0]);
if (!answer){
message = prompt(`please respond`);
/*do {
let answer = prompt(questions[i][0]);
}while(answer === null);*/
}
else if (answer === questions[i][1]){
console.log (`correct`);
counter++;
correct = [...questions[i][0]];
console.log(correct);
} else {
console.log (`wrong`);
wrong = [...questions[i][0]];
console.log(wrong);
}
}
function createList (arr) {
let item ='';
for (let i = 0; i < arr.length; i++){
item += `<l1>${arr[i]}</l1>`;
return item;
}
}
document.querySelector('main').innerHTML = `<h1>you got ${counter} correct</h1>
You got these questions right!
<ol>
${createList(correct)}
</ol>
You got these questions wrong!
<ol>
${createList(wrong)}
</ol>`;
1 Answer
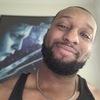
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi VICTORIA,
First off, a string is essentially an array of characters. So, const firstName = 'Brandon White'
is stored by the computer's memory like this: ['B', 'r', 'a', 'n', 'd', 'o', 'n', ' ' , 'W', 'h', 'i', 't', 'e']
, so when you say you want to spread questions[i][0]
(which is a string), you're basically saying, break up the string (ie. 'How many lines are in the sea?') by its individual characters and push each one into a new array. You could change your code to [...questions[i]]
, and then it would push the question and answer into the array (without breaking up the string), but I don't think that's what you want.
In this case, your best option would probably be to use the push method (ie. correct.push(questions[i][0]).
As far as your list items, I think the issue you may be running into is that in your createList function you're returning your item inside the for loop. If you aren't already aware, a return statement immediately exits its enclosing function (returning whatever value—if any—that's attached to it). It doesn't continue with any other code inside that same function, it simply exits. Because it's nested within your for loop, the only list item that's created before the return statement stops execution of the function is the first list item. But if you move that return statement to outside of the for loop (but within the same function), I believe you'll get the results you're looking for.
Great job getting this far. Keep it up, coder.
VICTORIA DENNIS
Full Stack JavaScript Techdegree Student 3,679 PointsVICTORIA DENNIS
Full Stack JavaScript Techdegree Student 3,679 PointsYou've got a really good eye! I didn't notice the return not actually being used in the function. And by using the push method I was able to make it all work, in my own way! Thanks a lot!