Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial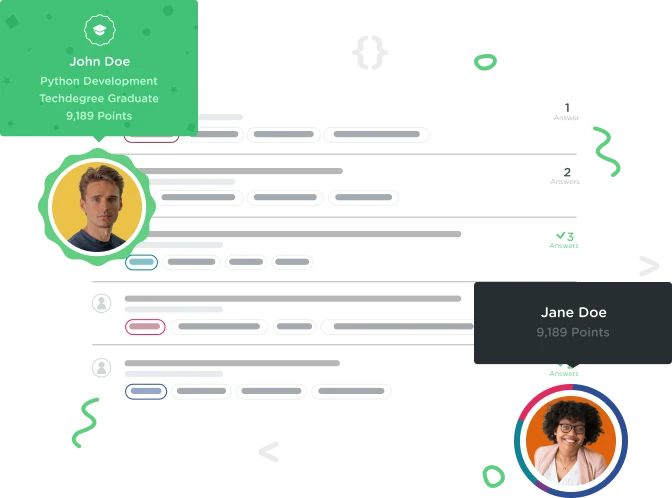

prat pandy
154 PointsCan you correct it ?
Can you tell me Where m i going wrong ?
struct Person {
let firstName: String
let lastName: String
}
func getFullName(firstname: String, lastname: String) -> String
{
return ("firstname" + "lastname")
}
}
var fullName1 = Person(firstname: "Bob", lastname: "Pandy")
1 Answer

Marie Nipper
22,638 PointsHey! You're pretty close. When you declare the instance function, you don't need to add arguments for this challenge.
func getFullName() -> String {
}
Now within the return statement, you're literally telling it to return "firstNamelastName". The literally words, not what they would compute to being. But you're close. To interpolate string/variable data like you're needing, you need to use the backslash paren syntax. And with this, you don't need a plus sign because the compiler will see the whitespace.
//In a return statement this would be "Justin Nipper"
let variableName = "Justin"
let anotherVariableName = "Nipper"
"\(variableName) \(anotherVariableName)"
//If you put the two variables you interpolated together without the space, it would return "JustinNipper"
"\(variableName)\(anotherVariableName)"
So to put your return statement together, it should look like this:
return "\(firstName) \(lastName)"
Now, the next issue you're going to have is the return statement you wrote evaluates "firstname" & "lastname" but the struct is defining "firstName" & "lastName". (note the camel case letters. It'll cause you another issue).
With all that, the code should finally look like this:
struct Person {
let firstName: String
let lastName: String
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}