Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial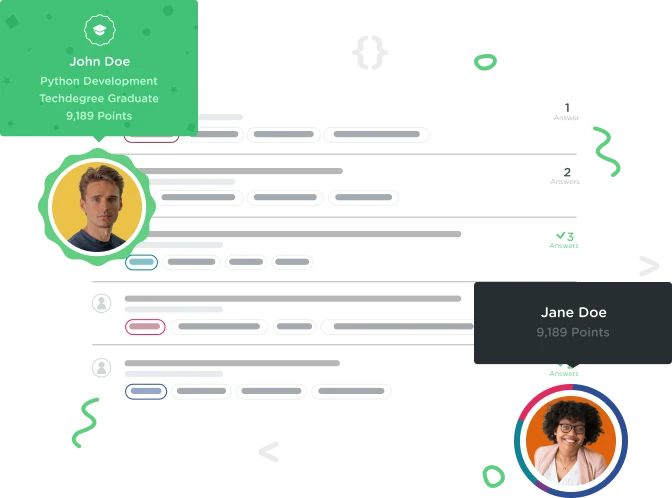
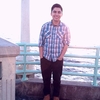
Cesar Marroquin
10,520 PointsCan you declare an array of unknown size in Javascript?
Can this be done? Will it expand as it gets filled up with elements, or would you just have to create a very large array that would be more than enough size for any data you might want.
3 Answers

Myroslav Tkachenko
10,581 PointsYes. Simply create an empty array:
var a = [];
or
var a = new Array();
Then you can add more elements dynamically by specifying any index (your array can even have "gaps" in it, an 'undefined' elements):
a[0] = "first";
a[2] = "third";
or simply add an element to the end of the array:
a[0] = "first";
a[a.length] = "next element";
The elements in an array may not be of the same type. As you can see, arrays in JavaScript are very flexible, so be careful with this flexibility :) There are a handful of methods to manipulate arrays like .pop(), .push(), .shift(), .unshift() etc.
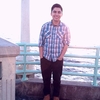
Cesar Marroquin
10,520 PointsThank you so much that was very helpful:)!

Myroslav Tkachenko
10,581 PointsYou are welcome! Glad to help.
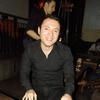
Davide Pugliese
4,091 PointsI think this in Java, that did not include this at the beginning like Javascript, is called an array list. Normally, you want to push this a step forward and create your own structures which are: stack, queue. http://www.i-programmer.info/programming/javascript/1674-javascript-data-structures-stacks-queues-and-deques.html http://en.wikibooks.org/wiki/Data_Structures/Stacks_and_Queues