Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial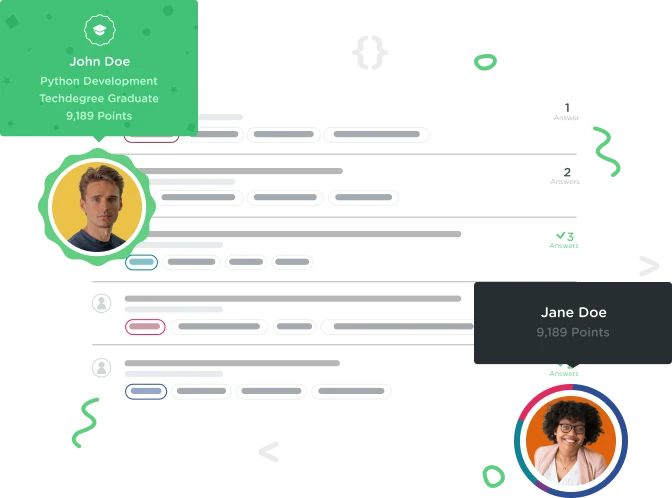

Robert Rajczyk
3,699 PointsCan you explain me what I am doing wrong?
Hello,
Can you please explain me what I am doing wrong and how I should correct it?
Best regards, Robert
def disemvowel(word):
vowels = [ "A", "a", "E", "e" , "I", "i", "O", "o", "U", "u"]
for letters in word:
if letters in vowels:
word.remove(letters)
return word
2 Answers

Umesh Ravji
42,386 PointsHi Robert,
The problem here is you are trying to call the remove method on a string. The string class doesn't have a remove method (strings in python are immutable). The best way would be to convert the word into a list, so you can then call remove on it.
Removing items in a list isn't always straightforward, and you should start from the end to ensure that all the items are removed.
def disemvowel(word):
word_list = list(word)
vowels = ["A", "a", "E", "e", "I", "i", "O", "o", "U", "u"]
for letter in reversed(word_list):
if letter in vowels:
word_list.remove(letter)
return ''.join(word_list)

Jason Anello
Courses Plus Student 94,610 PointsI would suggest iterating over a copy of the list. Your solution works here but might not be a good general pattern in situations where you're not necessarily removing all occurrences of a particular item.
As an alternative solution, you can avoid the list conversions and remove method by building up a new string of consonants using string concatenation.

Robert Rajczyk
3,699 PointsHello Umesh,
Thank you for answer - that all makes sense. However can you please explain to me in more details why we should start from the end? In my opinion if we are iterating each letter it should makes no difference.

Opanin Akuffo
4,713 PointsHow come this does not work in challenge but works on workspaces. Nothing seems wrong to me. Please help.
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
guy = list(word.lower())
for vowel in guy[:]:
if vowel in vowels:
guy.remove(vowel)
return ("".join(guy))

Jason Anello
Courses Plus Student 94,610 PointsIt's because you're lowercasing the entire word and not maintaining what was passed in.
You should either add the upper-case vowels to your list. Or lower-case the individual letters when doing your if
check.
Ryan Carson
23,287 PointsRyan Carson
23,287 PointsWhat error are you getting?