Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial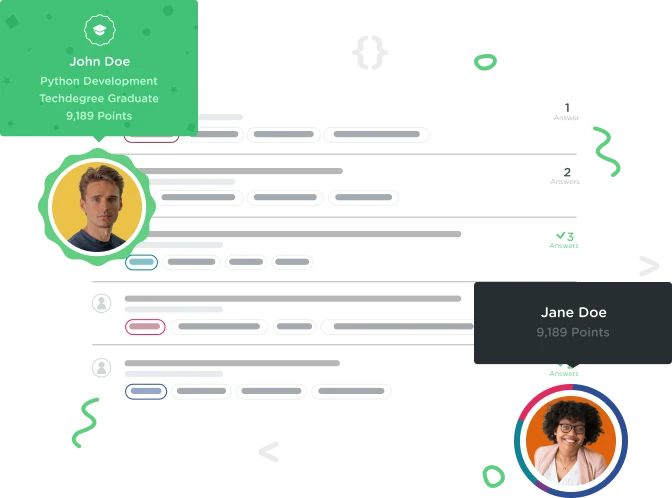

Eric Huang
519 PointsCan you have 2 Elif's in a row?
Everything works, like the fizz, buzz, not fizz or buzz, but "# If the number is divisible by both 3 and 5, print "is a FizzBuzz number." does not work. here is my code:
name = input("Please enter your name: ") number = int(input("Please enter a number: ")) print(name) print(number) if number %3 == 0: print("this is a fizz number") elif number %5 == 0: print("this is a buzz number") elif (number %5 == 0) and (number %3 == 0): print("this is a fizzbuzz number") else: print("this is neither a fizz number, or a buzz number")
1 Answer
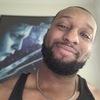
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Eric Huang,
You can have multiple elif
's in a row. The issue you're running into is one of logic though...
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
print(name)
print(number)
if number %3 == 0:
print("this is a fizz number")
elif number %5 == 0:
print("this is a buzz number")
elif (number %5 == 0) and (number %3 == 0):
print("this is a fizzbuzz number")
else: print("this is neither a fizz number, or a buzz number")
The reason "this is a fizzbuzz number" never prints is because of the order in which it comes. The thing about if...elif...else conditions is, if one condition is true, then the other conditions aren't even evaluated. This is where the logic error comes in. In order for a number to be a fizzbuzz number, it has to be divisible by both 3 and 5. As such, we take a number like 15. And we say, "Are you divisible by 3?" The answer is "Yes", and so "This is a buzz number" is printed to the console immediately, and the chain of if...elif...else statements is broken. The interpreter for python never even tests if it's divisible by both.
So... in order to check if a number is a fizzbuzz number, you need to either move the test for fizzbuzz to be the first one evaluated, or you need to add more specificity to the other tests (i.e. is number divisible by three while not being divisible by five).
Eric Huang
519 PointsEric Huang
519 PointsThanks Brandon! I understand now.