Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial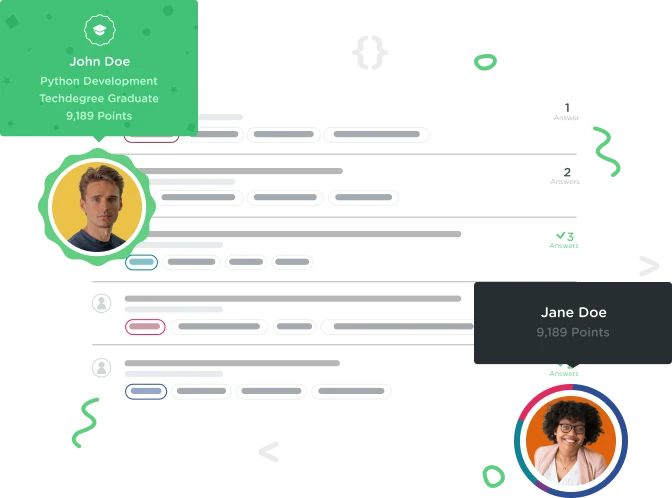
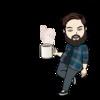
Philip Schultz
11,437 PointsCan you have multiple conditions in while loops?
I'm most familiar with the Java language and I'm trying to pick up on Python. In Java, you can have multiple conditions inside of while loops, but I can't figure out how to do it in Python. For example: I'm trying to do the extra credit assignment for the number game.(Try to build the opposite of this game. Make a game where the computer tries to guess your secret number. You'll need to come up with a way to tell the computer if it's too high, too low, or if it guesses the number!).
So, I want there to be a while loop in the program that allows the computer to guess a number 3 times or until the computer guesses the number correctly.
In java it would look something like this: while(!(correctGuess) || (attempts.length < 3) ){ code }
How would it look in Python?
Thanks for the help everyone!
2 Answers

andren
28,558 PointsYou definitively can.
The main differences is that Python does not use the !
and ||
symbols, opting instead to use words to represent these operations. Specifically not
is used instead of !
and or
is used instead of ||
.
So the while
loop you posted would look like this in Python:
while not correctGuess or len(attempts) < 3:
# Code goes here
As you might be able to guess the &&
symbol is not used either, being replaced instead with the word and
.
Edit: Forgot to mention that lists does not have a length
property. You have to use the built-in len
function to get the length. That is a somewhat universal thing in Python, classes are usually designed to work with len
instead of having their own properties or methods to access the length.
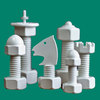
Steven Parker
230,274 PointsI think you may have meant "&&" instead of "||" to have that do what you describe.
So with that change, the Python equivalent would be:
while not correctGuess and len(attempts) < 3:
And while it's allowed, it would be unconventional to use camel case for variable names in Python.
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsThank you, Andren. I have one more question if you don't mind. Why doesn't this code work (below)? Shouldn't it stop looping as soon as either test or test_2 hits 0? For some reason, it works when I change the or to and, but that doesn't make sense to me because I want the loop to break when one 'OR' the other is false, not both. It should stop as soon as test_2 hits zero, right?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsThat's another example of what I pointed out about the other loop. The loop continues to run while the expression is "truthy", which is when the values are not 0. So to have the loop stop if either one becomes 0, you should combine them using "and":
while test and test_2:
Essentially, this means "continue looping while test and test_2 are both not zero".
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsHey Steven, Thank you for the response.