Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial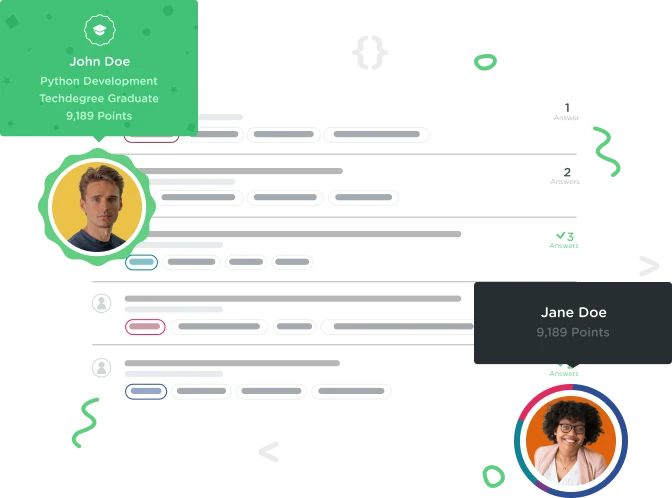

Justin Oswald
5,969 PointsCan you help me figure out this bug in a program I built??
the first part of code runs (regarding the subHeading), then it runs into a bug at line 11 when I try to type a color in the input text box. I wrote it identical to the block just above it (for the subHeading) so I am at a loss.
const subHeading = document.getElementById('subHeading')
const subHeadingButton = document.getElementById('subHeadingInputButton')
const subHeadingInput = document.getElementById('subHeadingInput')
subHeadingButton.addEventListener('click', () => {
subHeading.style.color=subHeadingInput.value
})
const banana = document.getElementById('listItem[0]')
const button1 = document.getElementById('1')
const input11 = document.getElementById('11')
button1.addEventListener('click', () => {
banana.style.color=input11.value
})
const sky = document.getElementById('listItem[1]')
const button2 = document.getElementById('2')
const input22 = document.getElementById('22')
button2.addEventListener('click', () => {
sky.style.color=input22.value
})
const firetruck = document.getElementById('listItem[2]')
const button3 = document.getElementById('3')
const input33 = document.getElementById('33')
button3.addEventListener('click', () => {
firetruck.style.color=input33.value
})
const frog = document.getElementById('listItem[3]')
const button4 = document.getElementById('4')
const input44 = document.getElementById('44')
button4.addEventListener('click', () => {
frog.style.color=input44.value
})
const grapefruit = document.getElementById('listItem[4]')
const button5 = document.getElementById('5')
const input55 = document.getElementById('55')
button5.addEventListener('click', () => {
frog.style.color=input55.value
})
const paper = document.getElementById('listItem[5]')
const button6 = document.getElementById('6')
const input66 = document.getElementById('66')
button6.addEventListener('click', () => {
paper.style.color=input66.value
})
const dory = document.getElementById('listItem[6]')
const button7 = document.getElementById('7')
const input77 = document.getElementById('77')
button7.addEventListener('click', () => {
dory.style.color=input77.value
})
const nemo = document.getElementById('listItem[7]')
const button8 = document.getElementById('8')
const input88 = document.getElementById('88')
button8.addEventListener('click', () => {
nemo.style.color=input88.value
})
const blood = document.getElementById('listItem[8]')
const button9 = document.getElementById('9')
const input99 = document.getElementById('99')
button9.addEventListener('click', () => {
blood.style.color=input99.value
})
const skin = document.getElementById('listItem[9]')
const button10 = document.getElementById('10')
const input1010 = document.getElementById('1010')
button10.addEventListener('click', () => {
skin.style.color=input1010.value
})
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<h2 id='subHeading'>Change this heading to what ever color you choose.</h2>
<button id='subHeadingInputButton'>Change Color</button>
<input id='subHeadingInput' type=text>
<ul id='listItems'>
<li>Banana</li> <button id='1'>Change Color</button> <input id='11' type=text>
<li>Sky</li><button id='2'>Change Color</button> <input id='22' type=text>
<li>Fire Truck</li><button id='3'>Change Color</button> <input id='33' type=text>
<li>Frog</li><button id='4'>Change Color</button> <input id='44' type=text>
<li>Grapefruit</li><button id='5'>Change Color</button> <input id='55' type=text>
<li>Paper</li><button id='6'>Change Color</button> <input id='66' type=text>
<li>Dory</li><button id='7'>Change Color</button> <input id='77' type=text>
<li>Nemo</li><button id='8'>Change Color</button> <input id='88' type=text>
<li>Blood</li><button id='9'>Change Color</button> <input id='99' type=text>
<li>Skin</li><button id='10'>Change Color</button> <input id='1010' type=text>
</ul>
<script src="app.js"></script>
</body>
</html>

Justin Oswald
5,969 PointsUncaught TypeError: Cannot read property 'style' of null at HTMLButtonElement.button1.addEventListener (app.js:11)
1 Answer

Leandro Botella Penalva
17,618 PointsHi Justin,
Since you don't have element with the id "listItem[X]" the document.getElementById returns null. You would need to set an ID individually to each list item to target it with javascript.
I also simplified your javascript code, it might be difficult to understand if you have a basic knowledge of javascript, but this will show you the power of JavaScript:
function changeColorToSibling() {
this.previousElementSibling.style.color = this.nextElementSibling.value;
}
const buttons = document.querySelectorAll("button");
for (const button of buttons) {
button.addEventListener("click", changeColorToSibling);
}
Hope it helps
Jesus Mendoza
23,289 PointsJesus Mendoza
23,289 PointsWhat error are you getting?